sort array
Arrays serve as valuable data structures in programming for grouping and storing similar data types as a single cohesive unit. Instead of creating multiple individual variables like arr1, arr2, arr3, and so on, arrays allow developers to use a single array variable, such as "numbers," and access its elements using indices, like arr[0], arr[1], arr[2], and so forth, to represent individual data points.
Each element within an array is accessed using an index, which is a numerical value that denotes the position of the element within the array. Indices typically start from 0, indicating the first element, and continue incrementally, correlating to the order of elements within the array.
Array sort - C# , VB.Net
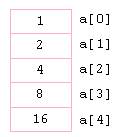
Using arrays not only simplifies variable management but also promotes efficient and organized data handling. By grouping related data together in a single array, operations like iteration, sorting, and searching become more straightforward and streamlined. This makes arrays an invaluable tool for managing large sets of data, especially when data elements share similar characteristics or are related to one another in some way.
Integer Array Example
C#String Array Example
C#Sorting Arrays
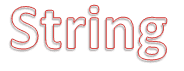
Arrays in programming provide the capability to sort their elements in both ascending and descending order, offering flexibility in data manipulation. The Array.Sort method is a powerful tool to achieve this functionality for one-dimensional arrays.
To sort an array in ascending order, you can simply call the Array.Sort method without any additional parameters. This method will rearrange the elements within the array, placing them in ascending order based on their natural ordering, determined by their data type.
How to sort an Integer Array
The following source code shows how to sort an integer Array in ascending order.
C#How to sort a String Array
The following code shows how to sort a string Array in ascending orde.
C#Sort Array in descending order
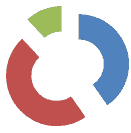
The following code demonstrates how to sort an array in ascending order, but similarly, you can arrange the elements in descending order as well. For this purpose, the Array.Reverse method can be employed, which effectively reverses the sequence of elements in a one-dimensional array. By utilizing Array.Reverse, developers can efficiently rearrange the elements in the opposite order, enabling the array to be sorted in descending fashion. This approach serves as a valuable complement to Array.Sort, expanding the array manipulation capabilities and allowing for seamless sorting operations in both ascending and descending directions.
Sort an Integer array in descending order
The following code shows how to sort an Integer array in reverse order.
C#Sort a String array in descending order
The following code shows how to sort a string array in reverse order.
C#LINQ OrderByDescending
To reverse the order of an array, you can utilize the LINQ OrderByDescending method, which effectively arranges elements in a descending manner. This method sorts the elements from the highest to the lowest values, offering a valuable solution for sorting arrays in a reverse order. The provided C# source code demonstrates how to achieve this by utilizing LINQ OrderByDescending, showcasing a clear and concise approach to efficiently sort arrays in descending fashion. By employing LINQ's powerful features, developers can streamline the sorting process and enhance code readability, ensuring a robust and elegant solution for array manipulation tasks.