Catch multiple exceptions at once: C#
The try{} block serves as a designated section of code where exceptions may potentially arise. Following the try block, a catch{} block is employed to handle any exceptions that occur within the associated try block. It is possible for a single try block to be accompanied by multiple catch blocks, each tailored to handle different types of exceptions. This approach allows developers to efficiently manage various exceptional scenarios. However, the utilization of multiple catch blocks can sometimes result in redundant and repetitive code, leading to code maintenance challenges and reduced code elegance. To address this concern and enhance code readability and maintainability, developers often seek alternative strategies, such as exception hierarchies and polymorphism, to consolidate exception handling and minimize redundancy.
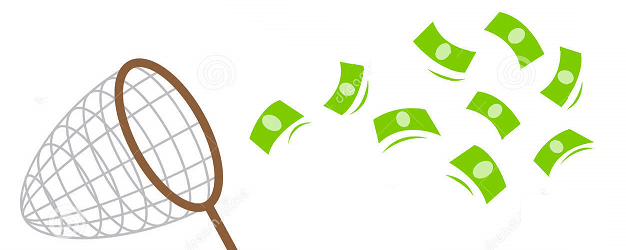
In the above example there is some code duplication and it is better to avoid while catching exception.
Switch on the types
You can avoid this code duplication by catch System.Exception and handle the specific types; otherwise, throw the exception to be handled higher up in the code:
C# Exception filters
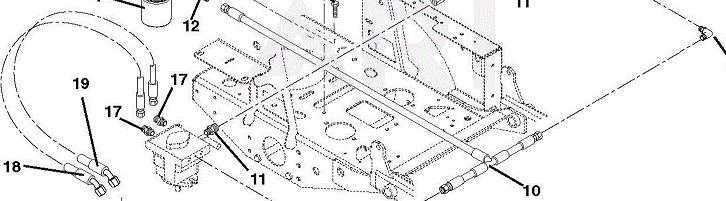
Using catch arguments and exception filters are valuable techniques to precisely filter and handle specific exceptions in C#. Exception filters, introduced in C# 6.0, provide a powerful mechanism for further evaluating exceptions to determine whether a particular catch clause should be applied or not. These filters act as conditional clauses that control the application of catch clauses based on the result of an expression evaluation. If the expression in the exception filter returns true, the associated catch clause executes its designated error-handling logic. Conversely, if the expression evaluates to false, the catch clause is bypassed, allowing the exception to propagate further or be handled by subsequent catch blocks. This flexibility enables developers to create catch blocks that selectively handle exceptions based on specific conditions, streamlining error management and improving code conciseness. By utilizing exception filters, developers can ensure that exception handling is fine-tuned to meet specific requirements, leading to more robust and maintainable code.
SyntaxHere the catch block will be entered if and only if ex.Code == 403. If the condition is not verified, the exception will bubble up the stack until it's caught somewhere else or terminates the process .
Thus, if we want to handle multiple types of exceptions in the same way using Exception Filters , we could write this: