Parse XML - XmlTextReader
XML, being a self-descriptive language, not only contains data but also provides the necessary rules for parsing and extracting the data it encapsulates. Parsing, which involves reading an XML file, entails the extraction of information found within the XML tags present in the document. There are multiple methods to parse or read an XML Document, and this chapter illustrates one of the simplest and most straightforward approaches for reading XML files.
XML Parser in C# and VB.Net
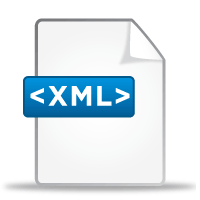
Parsing an XML file, developers gain access to the valuable data encapsulated within the XML tags while adhering to the defined rules and structure specified within the XML document. The parsing process facilitates efficient extraction and processing of relevant data, enabling seamless integration and utilization of the information stored in XML files.
Throughout this chapter, readers are introduced to an easily implementable method for reading XML files, providing a user-friendly solution that enhances comprehension and simplifies the parsing process. By utilizing this approach, developers can effectively interact with XML data, making it a valuable resource for various software applications and data manipulation tasks.
Parse XML with XmlTextReader in C# and VB.Net
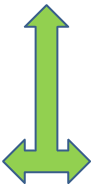