Datatype of a variable
Type Checking
The concept of "type" plays a crucial role as it pertains to data types. Within the .NET Runtime, a specialized type known as System.Type is defined, serving as a fundamental representation of various data types in the System. This System.Type diligently stores crucial type information within variables, properties, or fields. Remarkably, each class carefully crafted within the system is intrinsically associated with its own corresponding System.Type, bestowing upon it the capability to encapsulate and comprehend the underlying data type attributes.
Determine type of a variable
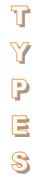
At runtime, every data type within the .NET framework is impeccably represented by an instance of System.Type. This invaluable System.Type object allows developers to access comprehensive information about the type, including its superclasses, methods, properties, fields, and more. Retrieving the System.Type object can be accomplished in two fundamental ways: firstly, by invoking the GetType method on a specific instance of an object; secondly, by utilizing the typeof operator followed by the name of the type. By employing either of these approaches, developers gain access to a wealth of essential details about the type at runtime, empowering them to perform dynamic and flexible operations within their applications.
typeof operator
The typeof operator serves as a valuable tool in obtaining the System.Type object for a specific data type in the .NET framework. This operator is commonly utilized as a parameter, variable, or field within the code. Its primary purpose lies in facilitating a compile-time lookup, wherein, given a symbol representing a class name, the corresponding Type object is retrieved. By utilizing the typeof operator, developers can efficiently access the metadata and essential information about the type during the compilation process. This feature enables static analysis and early binding, contributing to the robustness and efficiency of the code. The typeof operator proves invaluable in scenarios where the type information is required at compile time, empowering developers to make informed decisions and perform targeted operations within their applications.
C#