Textbox to allow only numbers C# VB.Net
The TextBox control serves to display or accept a single line of unformatted text. However, in various scenarios, it may be necessary to restrict input to numeric values.
How to: Create a Numeric Text Box
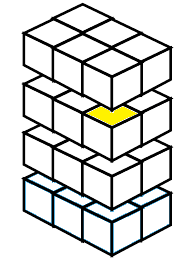
To achieve this, several useful techniques can be employed to ensure that the TextBox only accepts numbers as input, preventing invalid or unwanted characters from being entered. These techniques help enhance user experience, data validation, and the accuracy of numerical data entry in the TextBox control.
You can use Regular Expression to validate a Textbox to enter number only.
System.Text.RegularExpressions.Regex.IsMatch(textBox1.Text, "[ ^ 0-9]")
In this case your Textbox accept only numbers.
The following method also you can force your user to enter numeric value only.
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar))
{
e.Handled = true;
}
If you want to allow decimals add the following to the above code.
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.'))
{
e.Handled = true;
}
VB.Net
If (Not Char.IsControl(e.KeyChar) _
AndAlso (Not Char.IsDigit(e.KeyChar) _
AndAlso (e.KeyChar <> Microsoft.VisualBasic.ChrW(46)))) Then
e.Handled = True
End If
C# Source Code
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void textBox1_TextChanged(object sender, EventArgs e)
{
if (System.Text.RegularExpressions.Regex.IsMatch(textBox1.Text, " ^ [0-9]"))
{
textBox1.Text = "";
}
}
private void textBox1_KeyPress(object sender, KeyPressEventArgs e)
{
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.'))
{
e.Handled = true;
}
}
}
}
VB.Net Source Code
Public Class Form1
Private Sub TextBox1_KeyPress(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles TextBox1.KeyPress
If (Not Char.IsControl(e.KeyChar) _
AndAlso (Not Char.IsDigit(e.KeyChar) _
AndAlso (e.KeyChar <> Microsoft.VisualBasic.ChrW(46)))) Then
e.Handled = True
End If
End Sub
Private Sub TextBox1_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles TextBox1.TextChanged
If System.Text.RegularExpressions.Regex.IsMatch(TextBox1.Text, "[ ^ 0-9]") Then
TextBox1.Text = ""
End If
End Sub
End Class
NEXT..... NullReferenceException in C#