Top Python Interview Questions (2024)
What is Python?
Python is a versatile, high-level programming language known for its simplicity, readability, and extensive standard library. It supports multiple programming paradigms and is widely used in various fields, from web development to scientific computing.
What are the key features of Python?
Python's key features include dynamic typing, easy-to-understand syntax, automatic memory management, and a rich standard library. It supports object-oriented, functional, and procedural programming, making it adaptable to different coding styles.
What is Python good for?
Python is well-suited for a wide range of applications, including web development (with frameworks like Django and Flask), data analysis and visualization (with tools like NumPy and Matplotlib), scientific computing, automation, artificial intelligence, and more.
Is Python platform independent?
Yes, Python is platform independent. Code written in Python can be executed on various operating systems without modification, thanks to its interpreters available for different platforms.
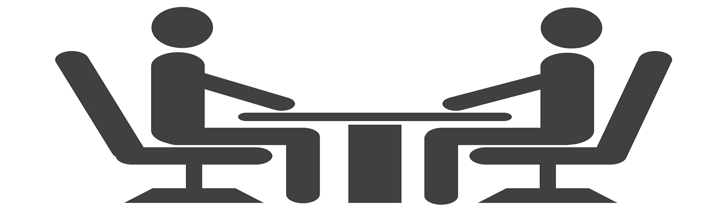
Is Python case-sensitive?
Yes, Python is case-sensitive. Variables, functions, and class names with different letter cases are treated as distinct entities.
What is Scope in Python?
Scope in Python defines the region where a variable is accessible. It can be local (within a function), global (across the module), or built-in (part of Python's standard library).
What is lambda in Python?
Lambda in Python is an anonymous function defined using the lambda keyword. It is often used for short, one-time operations where writing a full function is unnecessary.
What is self in Python?
In Python, self refers to the instance of a class. It is used within class methods to access attributes and methods of the instance itself.
What is slicing?
Slicing in Python involves extracting a portion of a sequence (like a list, string, or tuple) using the colon (:) notation. It provides a concise way to manipulate and retrieve parts of sequences.
What is a dynamically typed language?
Python is a dynamically typed language, meaning you don't need to declare variable types explicitly. The type of a variable is determined at runtime based on the assigned value.
What is init?
__init__ is a special method in Python classes, often referred to as the constructor. It is automatically called when a new instance of the class is created and is used to initialize attributes and perform setup operations.
How is memory managed in Python?
Memory management in Python is handled by its built-in memory manager. It employs techniques like reference counting and a garbage collector to manage memory allocation and deallocation, ensuring efficient memory usage.
Last Updated : 02 Jan 2024
- Python Interview Questions (Part 2)
- Python Interview Questions (Part 3)
- What is python used for?
- Is Python interpreted, or compiled, or both?
- Explain how python is interpreted
- How do I install pip on Windows?
- How do you protect Python source code?
- What are the disadvantages of the Python?
- How would you achieve web scraping in Python?
- How to Python Script executable on Unix
- What is the difference between .py and .pyc files?
- What is __init__.py used for in Python?
- What does __name__=='__main__' in Python mean?
- What is docstring in Python?
- What is the difference between runtime and compile time?
- How to use *args and **kwargs in Python
- Purpose of "/" and "//" operator in python?
- What is the purpose pass statement in python?
- Why isn't there a switch or case statement in Python?
- How does the ternary operator work in Python?
- What is the purpose of "self" in Python
- How do you debug a program in Python?
- What are literals in python?
- Is Python call-by-value or call-by-reference?
- What is the process of compilation and Loading in python?
- Global and Local Variables in Python
- Static analysis tools in Python
- What does the 'yield' keyword do in Python?
- Python Not Equal Operator (!=)
- What is the difference between 'is' and '==' in python
- What is the difference between = and == in Python?
- How are the functions help() and dir() different?
- What is the python keyword "with" used for?
- Why isn't all memory freed when CPython exits
- Difference between Mutable and Immutable in Python
- Python Split Regex: How to use re.split() function?
- Accessor and Mutator methods in Python
- How to Implement an 'enum' in Python
- What is Object in Python?
- How to determine the type of instance and inheritance in Python
- Python Inheritance
- How is Inheritance and Overriding methods are related?
- How can you create a copy of an object in Python?
- Class Attributes vs Instance Attributes in Python
- Static class variables in Python
- Difference between @staticmethod and @classmethod in Python
- How to Get a List of Class Attributes in Python
- Does Python supports interfaces like in Java or C#?
- How To Work with Unicode strings in Python
- Difference between lists and tuples in Python?
- What are differences between List and Dictionary in Python
- Different file processing modes supported by Python
- Python append to a file
- Difference Between Multithreading vs Multiprocessing in Python
- Is there any way to kill a Thread in Python?
- What is the use of lambda in Python?
- What is map, filter and reduce in python?
- Is monkey patching considered good programming practice?
- What is "typeerror: 'module' object is not callable"
- Python: TypeError: unhashable type: 'list'
- How to convert bytes to string in Python?
- What are metaclasses in Python?