VB.Net - Interview Questions and Answers
Visual Basic is a purpose-built programming language tailored for Windows programming, while VB.NET serves as the successor to the original Visual Basic (VB) language. It seamlessly integrates with the robust .NET Framework and the Common Language Runtime, affording exceptional language interoperability and heightened security measures. Below, we present a curated selection of VB.NET Interview Questions, focusing on the technology and development aspects of VB.NET. We urge you to allocate sufficient time to thoroughly review these common interview inquiries, as they are likely to be posed during your evaluations.
Difference Between Assembly and DLL?
An assembly serves as the fundamental building block for deploying .NET applications, representing a file encompassing essential elements such as the MSIL Code and Metadata. In the .NET environment, assemblies manifest in two extensions, namely .exe and .dll. While all .NET dlls are indeed assemblies, it is essential to recognize that not all assemblies are necessarily .dll files. Consequently, while it is correct to refer to a dll as an assembly, the reverse assertion may not always hold true, emphasizing the distinction between the two.
Can you use both C# and VB Project within a single Project?
No, you can't. It is not possible to intermix VB and C# code within the same project. During compilation, both languages will compile without raising any errors or complaints. The reason behind this is that a VB.NET project strictly compiles only the .vb files, while a C# project solely focuses on the .cs files. Consequently, any files in the project that do not correspond to the project's primary language will be effectively ignored during compilation, leading to the absence of errors. However, it is entirely feasible to utilize both C# and VB projects within a single solution, where you can have one project in VB and another in C#.
Is VB really case insensitive?
VB is mostly case insensitive, but there are exceptions. For example, XML literals and comprehension is case sensitive.
What is the purpose of the Async keyword in VB.NET?
Async methods are designed to facilitate non-blocking operations, ensuring that the execution flow can proceed without waiting for the asynchronous operation to conclude. In VB.Net, the "Async" keyword plays a crucial role in denoting that a lambda expression or method has asynchronous behavior. Consequently, such methods are appropriately referred to as async methods. Importantly, when a caller invokes an async method, it gains the advantage of resuming its tasks promptly, free from the obligation to await the completion of the async method's execution.
Is there a lock statement in VB.NET?
Yes. The SyncLock statement is the equivalent of C#'s lock statement in Vb.Net.
The lock statement ensures that one thread does not enter a critical section of code while another thread is in the critical section. If another thread tries to enter a locked code, it will wait, block, until the object is released.
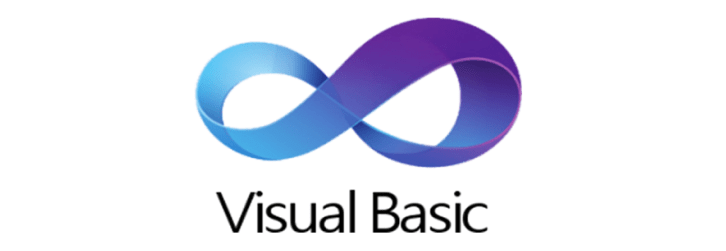
Difference between DirectCast() and CType() in VB.NET
DirectCast is more restrictive than CType . With CType() you can write something like Ctype("string",Integer). But with DirectCast the above statement would give a compile time error.
What is the equivalent VB keyword for 'break'?
In both Visual Basic 6.0 and VB.NET you would use:
- Exit - For to break from For loop.
- Exit Sub - For to break from Sub.
- Exit Function - For to break from Function.
What is the use of Option explicit?
When the "Option Explicit On" or "Option Explicit" settings are present in a file, it mandates the compulsory declaration of variables using the Dim or ReDim statements. Conversely, when the "Option Explicit" setting is set to OFF, variables can be utilized without the requirement for prior declaration. This distinction in behavior ensures a more robust and predictable coding environment when "Option Explicit On" is enabled, as it enforces explicit variable declarations, promoting cleaner and more maintainable code.
What is the use of shared variables in vb.net?
The "Shared" variable in VB.NET corresponds to the "static" variable in C#. This signifies that every instance of the class shares the same copy of the variable, property, or method. By employing the "Shared" keyword, you can ensure that a single instance of the variable exists throughout your entire application, thereby facilitating data sharing between objects of the class, rather than each object maintaining its separate copy. This design approach enhances memory efficiency and promotes a unified data state across the class instances.
What is the difference between And and AndAlso in VB.NET?
The And operator evaluates both sides, where AndAlso operator evaluates the right side if and only if the left side is true.
exampleHow to VB.NET Single statement across multiple lines
You can use the line-continuation character (_), at the point at which you want the line to break.
What is the equivalent for C#'s '??' operator in VB.NET
The IF() operator is the equivalent for C#'s '??' operator in VB.NET.
The If() operator can be called with three arguments or with two arguments.
How do you declare a Char literal in Visual Basic .NET?
A character literal is entered using a single character string suffixed with a C.
How to check for a Null value in VB.NET
The equivalent of null in VB is Nothing: