C# Interview Questions
C# stands as an exemplary, uncomplicated, and cutting-edge programming language, carefully crafted by the esteemed Microsoft Corporation. This state-of-the-art tool embraces a general-purpose approach, catering to a wide range of software development needs. In the subsequent segment, we have diligently compiled a comprehensive collection of major C# interview inquiries and their corresponding answers. This compilation has been thoughtfully designed to assist aspiring beginners as well as seasoned professionals in securing the ideal employment opportunity that aligns with their expertise and aspirations.
Explain the C# code execution process?
Compiler time process:- C# Source Code >>> .Net Compiler >>> Byte Code (MSIL + META DATA)
- Byte Code (MSIL + META DATA) >>> Just-In-Time (JIT) Compiler >>> NATIVE CODE
C# code execution life cycle diagram is shown as follows :
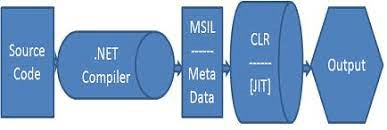
How do you force your .NET application to run as administrator?
You have to modify the manifest that gets embedded in the program, which will cause Windows (7 or higher) to always run the program as an administrator .
- Project>>>Add New Item, select "Application Manifest File".
Change the
This works on Visual Studio 2008 and higher.
What are the differences between Object and Var?
In C#, every object is derived from the Object type, whether directly or indirectly. Objects of this nature are compile-time variables, which necessitate boxing and unboxing operations for conversions, resulting in a potential decrease in performance. Additionally, due to limited type information available to the compiler, Object can accommodate various kinds of values, as it serves as the fundamental class within the .NET framework.
On the other hand, Var, another compile-time variable in C#, offers distinct advantages. It eliminates the need for boxing and unboxing operations since all type checking occurs during compilation. Once Var has been assigned a value, its type cannot be changed, ensuring type-safety and preventing potential runtime issues. Var can store values of any type, but it is mandatory to initialize Var variables at the time of declaration, ensuring clarity and accuracy in code execution.
What is a NullReferenceException
The occurrence of a NullReferenceException in programming signifies an attempt to utilize a reference variable that possesses a value of Nothing or null. This situation arises when the reference variable lacks an actual reference to an existing object instance within the memory heap.
Above code throws a NullReferenceException at the second line because you can't call the instance method ToUpper() on a string reference pointing to null.
Why is it wrong to specify access modifiers for methods interfaces?
Interfaces serve the fundamental purpose of delineating public Application Programming Interfaces (APIs). They inherently lack any alternative function for the methods contained within, aside from the potential for overriding them in derived classes. Consequently, any method (or static member) declared within an interface is automatically designated as public by definition. Hence, specifying such methods within an interface while entrusting their implementation to subclasses or unrelated clients would result in an incomplete type definition. To enforce the necessity for overriding a specific set of private methods, it is advisable to declare an abstract class encompassing a collection of abstract protected methods.
How to check that a nullable variable is having value?
Use the HasValue property of the Nullable
If the HasValue property is true, the value of the current Nullable
Why this error message: Cross-thread operation not valid
In the scope of user interfaces, a designated UI thread takes on the responsibility of generating UI elements and actively engaging with events such as mouse clicks and key presses. It is crucial to acknowledge that exclusive access to UI elements is limited solely to the UI thread. The System.Windows.Forms.Control class, along with its subclasses, can only be accessed through this singular thread. Consequently, due to the presence of a solitary thread, all UI-related operations are carefully queued as work items within this particular thread. Any endeavor to access members of the System.Windows.Forms.Control class from a thread distinct from the UI thread will inevitably result in a cross-thread exception.
Difference between object type and dynamic type variables?
Object types in C# have the flexibility to receive values from a wide range of sources, including value types, reference types, as well as both predefined and user-defined types. However, it is essential to note that the compiler possesses limited knowledge about the specific type involved.
On the other hand, dynamic types exhibit similarities to object types in their ability to hold various values. However, unlike object types, dynamic type variables undergo type checking at runtime rather than compile time. This characteristic renders dynamic types less type-safe, as the compiler lacks explicit information regarding the type of variable involved.
What is safe and unsafe code in C#?
The main difference in C# is that a safe code is the one that executes under the supervision of the Common Language Runtime (CLR). ; and, an unsafe code is code that executes outside the context of the CLR.
In safe code, the CLR is responsible for various tasks, like:
- Managing memory for the objects.
- Performing type verification.
- Performing garbage collection.
While in unsafe code, a programmer is responsible for:
- Calling the memory allocation function.
- Making sure that the casting is done right.
- Making sure that the memory is released when the work is done.
Why this error message?
No connection could be made because the target machine actively refused it.
The occurrence of this error can be attributed to the absence of a server actively listening at the designated hostname and port. Essentially, it indicates that the target machine exists but lacks any services awaiting connections on the specified port. Consequently, establishing a connection becomes unfeasible. Typically, this situation arises due to potential obstacles hindering the connection, such as a firewall impeding access or the hosting process failing to listen on the designated port. The root causes could involve the service not running altogether or the service listening on a different port than expected.
Difference Between Select and SelectMany?
The Select operation serves as a straightforward mapping mechanism that transforms each source element into a corresponding result element in a one-to-one fashion. On the other hand, SelectMany is employed when multiple from clauses are present within a query expression, generating a new sequence by utilizing each element from the original sequence. Essentially, SelectMany facilitates the flattening of a sequence wherein each element of the sequence is considered distinct and separate.
Why this exception:
New transaction is not allowed because there are other threads running in the session
The occurrence of this exception can be attributed to Entity Framework's implicit transaction mechanism, which is triggered when invoking the SaveChanges() method. This method initiates a transaction that automatically rolls back any changes made to the database if an exception arises before the completion of the iteration. Conversely, if the iteration completes without exceptions, the transaction commits, ensuring data persistence. To address this issue effectively, it is advisable to adopt an alternative pattern that avoids saving while in the process of reading data or explicitly declare a transaction to manage the operations more explicitly.
When should reflection be used?
Reflection encompasses a valuable capability that allows for the dynamic evaluation of information and execution of code during runtime. It empowers developers to inspect assemblies, types, and members, enabling the dynamic invocation of methods within an assembly. This feature holds great significance within the context of .NET languages, known for their strong typing. The ability to access metadata through reflection proves to be exceptionally useful, offering a versatile approach to achieving dynamic behavior. While its usage is not mandatory, it presents a highly advantageous tool for developers seeking flexible and adaptable programming solutions.
Advantage of Immutable String in C# programming
When you try to modify a string object in C#, a new object will be created. The original string will remain untouched. This technique is called immutability.
Advantages:- Immutable strings are cheap to copy, because you don't need to copy all the data - just copy a reference or pointer to the data.
- Immutable strings simplify multithreaded programming since reading from a type that cannot change is always safe to do concurrently.
- Immutable strings allows for a reduction of memory usage by allowing identical values to be combined together and referenced from multiple locations.
- Immutable strings eliminate the need for defensive copies, and reduce the risk of program error.
- Immutable strings simplify the design and implementation of certain algorithms because previously computed state can be reused later.
Why this error message:
The Controls collection cannot be modified because the control contains code blocks
The presence of <%...%> code blocks within your Page.Header, potentially within a master page, initiates processing during the page rendering phase rather than within the code-behind or page events. Consequently, when attempting to add an item to the Controls collection of said control, an error message is encountered. To resolve this issue, it is recommended to modify the code block by initiating it with < %# instead of < %=. This adjustment transforms the code block from a Response.Write code block to a databinding expression, as <%# ... %> databinding expressions do not fall under the category of code blocks. By adopting this approach, the Common Language Runtime (CLR) no longer raises any objections or errors.
How do you generate a stream from a string?
You can use the MemoryStream class , calling Encoding.GetBytes to turn your string into an array of bytes first.
What is Kestrel?
Kestrel, a comprehensive web server, comes prepackaged as a core component in ASP.NET Core project templates. It provides a robust foundation for hosting web applications. Kestrel can be utilized as a standalone server or in conjunction with a reverse proxy server, such as IIS, Nginx, or Apache. When integrated with a reverse proxy server, the latter receives incoming HTTP requests from the Internet and performs initial processing before forwarding them to Kestrel for further handling and execution. This collaboration between Kestrel and a reverse proxy server optimizes performance and enhances security in web application deployments.

What are the different states of a Thread in C#?
The various states of a Thread in C# include:
- Unstarted State - Instance of the thread is created but the start() method is not called.
- Ready State (Runnable) - The thread is ready to run and waiting CPU cycle.
- Running State - A thread that is running.
- Not Runnable State - The thread is in not runnable state, if sleep() or wait() method is called on the thread, or input/output operation is blocked.
- Dead State(Terminated) - Thread enters into dead or terminated state.
The request was aborted: Could not create SSL/TLS secure channel
The encountered error is of a generic nature, and it can stem from various factors that may lead to the failure of SSL/TLS negotiation. To address this, it is essential to consider the ServicePointManager.SecurityProtocol property, which facilitates the selection of the appropriate version of the Secure Sockets Layer (SSL) or Transport Layer Security (TLS) protocol for establishing new connections. It is important to note that modifying the ServicePointManager settings should be done prior to creating the HttpWebRequest, as adjustments made afterwards will not take effect. Additionally, to resolve this issue, it may be necessary to enable other security protocol versions, expanding the range of supported protocols to ensure successful negotiation.
If you create HttpWebRequest before the ServicePointManager settings it will fail and shows the error message.
How to get the URL of the current page in C#?
The HttpContext Class serves as a comprehensive container encompassing all HTTP-specific details pertaining to an individual HTTP request. It offers seamless access to crucial properties such as Request, Response, and Server, enabling developers to interact with these intrinsic elements effectively. Notably, the AbsoluteUri property within the HttpContext class provides a holistic representation of the entire Uniform Resource Identifier (URI) contained within the associated Uri instance. This encompasses all components, including fragments and query strings, facilitating a comprehensive understanding and manipulation of the request's URI.
Why this error message: Unable to access the IIS Metabase
This error message says that Visual Studio devenv.exe is not running with sufficient privileges to access the IIS process. There are two solutions fo this error:
- Run VS as an Administrator and reopen the solution/project.
- Edit the web application's project file with a text editor and change this line from True to False:
The above changes will stop it using IIS and demanding higher privileges.
Why this exception: System.Net.Sockets.SocketException
A SocketException is raised by the Socket and Dns classes to indicate an error encountered during network operations. Typically, these exceptions arise from connectivity issues, often stemming from disparities in IP protocols (such as IPV4/IPV6) between the communicating servers or computers. Additionally, they can arise due to the presence of additional authentication rules configured on one of the involved computers, which affect inbound and outbound connectivity. These exceptions serve as indicators for developers to diagnose and address network-related errors, enabling them to establish successful communication between systems.
Possible causes for the error:
- Wrong IP address.
- Wrong port.
- Firewall blocking the connection.
- C# Interview Questions (part-1)
- C# Interview Questions (part-2)
- C# Interview Questions (part-3)
- Difference between a Debug and Release build
- Difference between normal DLL and .Net DLL
- What is an Interface in C#
- Difference between Abstract Class and Interface in C#
- Difference between a thread and a process
- Delegates in C# with Examples
- Differences between a control and a component
- Differences between Stack and Heap
- What is .Net Reflection
- Globalization and Localization | C#
- What is .Net serialization
- Difference between web service and .net remoting
- Difference between managed and unmanaged code
- Difference between Shallow copy and Deep copy
- Use of System.Environment Class
- What is the difference between private and shared assembly?
- Does the .NET have in-built support for serialization?
- How to properly stop the Thread in C#?
- Why are there five tracing levels in System.Diagnostics.TraceSwitcher?
- Why is XmlSerializer so slow?
- How many types of Jit Compilers?