ASP.NET Core MVC Interview Questions
ASP.NET Core MVC is a comprehensive and feature-rich framework designed for constructing robust web applications and APIs, utilizing the widely adopted Model-View-Controller (MVC) design pattern. This framework offers a patterns-based approach to web development, empowering developers to create dynamic websites with a clear separation of concerns.
With ASP.NET Core MVC, developers gain full control over the generated markup, allowing for precise customization and tailoring of the user interface. The framework also prioritizes test-driven development (TDD), enabling developers to easily write unit tests and ensure the reliability and maintainability of their codebase.
Furthermore, ASP.NET Core MVC keeps up with the latest web standards, ensuring compatibility and adherence to industry best practices. It utilizes modern technologies and conventions to provide a cutting-edge development experience.
ASP.NET Core MVC, the provided set of interview questions focuses on assessing candidates' knowledge and proficiency in ASP.NET Core MVC technologies and development practices. These questions serve to evaluate candidates' understanding of key concepts and their ability to apply them effectively in real-world scenarios.
What is ASP.NET Core?
ASP.NET Core, the open-source counterpart of Microsoft ASP.NET, offers developers the ability to build and deploy web applications that are platform-independent, running seamlessly on Windows, Mac, and Linux operating systems. This framework is designed with a modular architecture, ensuring minimal overhead and providing developers with the flexibility to construct solutions tailored to their specific needs. Notably, ASP.NET Core allows for multiple versions to coexist concurrently on the same server, granting the freedom to adopt the latest version for one application while keeping other applications running on their tested and stable versions. This flexibility and compatibility enable developers to effectively manage their application ecosystem and seamlessly transition to newer versions of ASP.NET Core as they become available.
What are some benefits of ASP.NET Core over the classic ASP.NET?
ASP.NET Core, introduced by Microsoft, represents an updated version of ASP.NET that brings along significant enhancements. While both project templates utilize the Full .NET Framework, there are notable distinctions in their functionality and architectural approach. The ASP.NET Web Application template caters to projects based on the legacy version of ASP.NET MVC, offering compatibility with the Global.asax file for handling application lifecycle events. In contrast, the ASP.NET Core Web Application template introduces a novel concept, encompassing an entirely new approach. It adopts the use of the wwwroot folder for static files, utilizes task runners for managing build processes, and embraces the power of OWIN middleware for seamless request handling and application flow. This modernized paradigm offers developers an improved development experience, enhanced performance, and greater flexibility in building web applications.
Main differences:- Asp.Net Build for Windows only while Asp.Net Core Build for Windows, Mac and Linux.
- Asp.Net Supports WebForm, Asp.Net MVC and Asp.Net WebAPI, whereas Asp.Net Core does not support WebForm. It supports MVC, Web API and Asp.Net Web pages originally added in .Net Core 2.0.
- Asp.Net support C#, VB and many other languages and also support WCF, WPF and WF while Asp.Net Core support only C#, F# language.
- You need to re-compile after the code change in Asp.Net while in ASP.NET Core browser will compile and executed the code and no need for re-compile.
How to explain OWIN and Katana in simple words?
OWIN (Open Web Interface for .NET) is an established standard, known as the OWIN Specification, while Katana is a .NET library developed by Microsoft. The primary purpose of OWIN is to establish a uniform interface between .NET web servers and web applications. By introducing an abstraction layer, OWIN aims to decouple web applications from specific web servers, enabling the seamless deployment of the same application across multiple web servers that support OWIN. Katana, built upon the OWIN specifications, encompasses a collection of components provided by Microsoft. These components include Web API, ASP.NET Identity, and SignalR, among others. Using the power of OWIN, Katana facilitates the development and integration of these components into web applications, ensuring compatibility and adherence to the established OWIN standards.
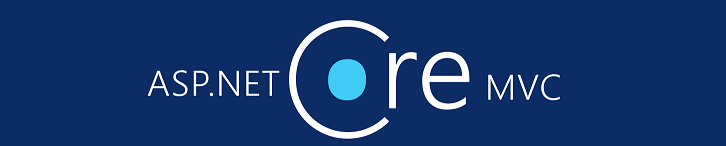
Can ASP.NET Core work with the .NET framework?
Yes. ASP.NET Core, being an officially supported framework by Microsoft, seamlessly integrates with the .NET framework. One notable advantage of utilizing the Full .NET framework in conjunction with ASP.NET Core is the accessibility of a wide range of well-established libraries and frameworks that have been primarily developed to target earlier versions of the .NET framework. These mature libraries and frameworks bring a wealth of functionality and proven solutions, allowing developers to use existing code and resources, streamline development efforts, and benefit from the extensive ecosystem that has evolved around previous versions of .NET. This compatibility ensures that developers can use the power and versatility of the Full .NET framework while capitalizing on the wealth of established tools and components that are readily available for their ASP.NET Core applications.
Why this error: A potentially dangerous Request.Form value was detected from the client
The occurrence of this error is attributed to the presence of HTML tags within your POST request. This situation serves as an indicator of a potential cross-site scripting (XSS) attack, prompting Asp.net to prohibit it as a default security measure. To mitigate this issue, it is recommended to perform HTML encoding at the specific points where certain characters might pose a risk due to their transition into a different sub-language with distinct contextual meanings.
The solution for resolving this error involves two options. Firstly, you can opt to HTML encode the data before submitting it, ensuring that any potentially harmful characters are transformed into their encoded counterparts. This approach maintains data integrity while effectively mitigating the XSS vulnerability. Alternatively, if you choose to disable the warning, it is essential to acknowledge the potential consequences, as this action may expose your application to XSS attacks. It is crucial to exercise caution and implement additional security measures to compensate for the disabled warning, should you decide to pursue this route.
Which protocol is used to call web service?
SOAP (Simple Object Access Protocol) serves as the protocol of choice when it comes to exchanging data in web services. It uses the XML information set as the standardized format for structuring messages, and relies on application layer protocols like HTTP or SMTP to facilitate the negotiation and transmission of these messages. SOAP provides a robust and interoperable means of communication between diverse systems, enabling seamless integration and data exchange in distributed environments. By utilizing the widely supported XML format and using existing application layer protocols, SOAP ensures a reliable and versatile framework for exchanging data in web services.
Explain the Cross page posting and Redirect Permanent in ASP.Net?
Cross-page posting refers to the practice of submitting form data to a different page, as opposed to the default behavior of posting data back to the same page in ASP.NET. This approach proves beneficial in scenarios where there is a need to transmit data to another page without the unnecessary overhead of reloading the current page solely for the purpose of redirecting the user to a different page via an HTTP 302 response (such as using Response.Redirect).
In web development, a Redirect Permanent is a method of achieving a permanent redirection from the requested URL to a specified URL. This process involves returning a 301 moved permanently response, indicating that the requested URL has permanently moved to the designated URL. By utilizing a Redirect Permanent, developers can effectively manage changes in URLs, ensuring that users and search engines are redirected to the correct and updated location of the desired content.
Explain how HTTP protocol works?
- First, the Browser looks up the destination server. If requested object is in DNS cache, it uses that information. Otherwise, DNS querying is performed until the destination server's IP address is found.
- Then, your browser opens a TCP connection to the destination server and sends the request according to Hypertext Transfer Protocol (HTTP). This step is much more complex with HTTPS.
- The server looks up the required resource (if it exists) and responds using HTTP protocol, sends the data to the browser. Browser receives HTTP response and may close the TCP connection, or reuse it for another request.
- The browser then uses HTML parser to re-create document structure which is later presented to you on screen. If it finds references to external resources, such as pictures, css files, javascript files, these are delivered the same way as the HTML document itself or offers a download dialog for unrecognized types.
What is MVC?
The Model-View-Controller (MVC) is a widely adopted software design pattern utilized for constructing user interfaces. It effectively partitions the program logic into three interconnected elements: the model, responsible for managing the data; the view, focused on presenting the user interface; and the controller, serving as the mediator for application logic. Each of these components is purposefully designed to handle specific development aspects within an application, promoting separation of concerns and maintainability.
In web development using the MVC pattern, incoming requests are routed to a controller component, which serves as the application logic orchestrator. The controller interacts with the model, using its functionalities to execute actions or retrieve data. After processing the request, the controller selects an appropriate view to present to the user, supplying it with the necessary data from the model. The view then renders the final page based on the provided data, culminating in the user interface presented to the user.
By adhering to the MVC pattern, developers can achieve a clear separation of responsibilities, promoting code reusability, testability, and maintainability. This architectural approach facilitates the development of scalable and organized applications by assigning distinct roles to each component, ensuring a structured and efficient user interface construction process.
What is the difference between ASP.NET Webforms and ASP.NET MVC?
ASP.NET Web Forms and MVC are two web frameworks developed by Microsoft. Each of these web frameworks offers advantages/disadvantages - some of which need to be considered when developing a web application.
- Asp.Net Web forms doesn't require much prior knowledge of HTML, JavaScript and CSS while Asp.Net MVC requires detailed knowledge of HTML, JavaScript and CSS.
- Asp.Net Web Form follow a traditional event-driven development model whereas Asp.Net MVC is a lightweight and follows MVC (Model, View, Controller) pattern based development, model.
- Asp.Net Web Form supports view state for state management at the client side while Asp.Net MVC does not support view state.
- Asp.Net Web Form follows Web Forms Syntax while Asp.Net MVC follow customizable syntax (Razor as default).
- Asp.Net Web Form has User Controls for code re-usability whereas Asp.Net MVC has Partial Views for code re-usability.
- In Asp.Net Web Form, Web Forms(ASPX) i.e. views are tightly coupled to Code behind(ASPX.CS) i.e. logic while in Asp.Net MVC, Views and logic are kept separately.
- Finally, Asp.Net Web Form is not Open Source while Asp.Net Web MVC is an Open Source.
How to enable Cross-Origin Requests (CORS) in ASP.NET Core?
You have to configure a CORS policy at application startup in the ConfigureServices method.
The CorsPolicyBuilder in builder allows you to configure the policy to your needs. You can now use this name to apply the policy to controllers and actions:
What is dependency injection?
Dependency Injection is a powerful concept that enables an object to fulfill its dependencies by receiving them from external sources. In software development, when a component relies on external resources to achieve its intended functionality, it becomes essential to establish knowledge of these resources, their location, and the means to interact with them.
By embracing Dependency Injection, dependent objects can be created externally to a class and subsequently provided to the class in various ways. The object receiving the dependencies is commonly referred to as the client, while the passed-in object is recognized as the service. The responsible code that supplies the service to the client is known as the injector. Rather than the client explicitly specifying which service it requires, the injector determines and communicates the appropriate service for the client's needs. The term "injection" signifies the act of delivering a dependency, represented by the service, into the client that utilizes it.
One significant benefit of Dependency Injection is its facilitation of easier testing. The injection of dependencies can be achieved through constructor-based injection, allowing for more straightforward and isolated unit testing. By decoupling dependencies from the client code, mock or test-specific implementations can be easily provided during testing, resulting in improved testability and overall code quality.
What is In-memory cache ?
ASP.NET Core introduces the capability to efficiently cache data within applications, enhancing performance and responsiveness. One of the caching options available in ASP.NET Core is In-Memory Caching, which utilizes memory storage on the web server to store cached data. This feature is implemented as a service and can be seamlessly incorporated into your application through dependency injection, utilizing the IMemoryCache interface instance within the constructor to enable the In-Memory Caching service provided by ASP.NET Core.
In scenarios where applications are distributed across multiple servers, it is crucial to ensure session stickiness when utilizing in-memory cache. Session stickiness ensures that subsequent client requests are consistently redirected to the same server, preserving the integrity of cached data and avoiding potential inconsistencies that may arise when serving requests from different servers.
By using in-memory caching in ASP.NET Core and implementing session stickiness in multi-server environments, developers can optimize the performance of their applications and ensure reliable data access, ultimately enhancing the overall user experience.
How to enable the in-memory cache in ASP.NET Core project?
You can enable the in-memory cache in the ConfigureServices method in the Startup class as shown in the code snippet below.
How to prevent Cross-Site Scripting (XSS) in ASP.NET Core?
Cross-Site Scripting (XSS) represents a significant security vulnerability that allows malicious actors to inject client-side scripts, typically JavaScript, into web pages. This form of attack operates by deceiving the targeted application into incorporating a
- Asp.Net Interview Questions (Part-1)
- Asp.Net Interview Questions (Part-2)
- Advantages of ASP.NET Web Development
- What is IIS - Internet Information Server
- What is Virtual Directory
- What is HttpHandler
- Page Directives in Asp.Net
- What is a postback
- What is IsPostBack
- What is global.asax
- Difference between Machine.config and web.config
- Difference between HTML control and Web Server control
- What is Query String
- Difference between Authentication and Authorization
- How to secure Connection Strings
- What is ASP.Net tracing
- Passing values between Asp.Net pages
- Differentiate between client side validation and server side validation
- How to Get host domain from URL
- Adding a Favicon To Your Website
- Asp.Net Textbox value in Javascript
- AutoEventWireup attribute in ASP.NET
- Can I use multiple programming languages in a ASP.net Web Application?
- Difference: Response.Write and Response.Output.Write
- How many web.config files can I have in an application?
- What is Protected Configuration in asp.net?
- Static variablesin .Net , what is their life span?
- Difference between ASP Session and ASP.NET Session?
- What does mean Stateless in Asp.Net?
- What is the Difference between session and caching?
- What are different types of caching using cache object of ASP.NET?
- Which method is used to remove the cache object?
- How many types of Cookies are available in ASP.NET?
- What is Page Life Cycle in ASP.net?
- What is the code behind and Inline Code in Asp.Net?
- What is master page in ASP.NET?
- Can you change a Master Page dynamically at runtime?
- What is cross-page posting in ASP.NET?
- How to redirect a page in asp.net without performing a round trip ?
- How to register custom server control on ASP.NET page?
- How do you validate Input data in Asp.Net?
- What's the difference between ViewData and ViewBag?
- Difference between Response.Redirect and Server.Transfer
- What is the function of the CustomValidator control?
- Define RequiredFieldValidator?
- Difference between custom control and user control
- Difference between Label and Literal control in ASP.Net
- What are the major events in Global.Asax file?
- What is Event Bubbling in asp.net ?
- What is Delay signing?
- What is the difference between in-proc and out-of-proc?
- What is the difference between POST and GET?
- A potentially dangerous Request.Form value was detected from the client