How to Create an Overlay Effect?
An overlay refers to a layer placed on top of another element or content to achieve a certain visual effect or functionality. Overlays can be created using HTML, CSS, and JavaScript to enhance the appearance or behavior of a webpage or graphical interface.
There are various types of overlays, each serving a different purpose:
- Image Overlay: An image overlay is a technique where one image is placed on top of another. This is often used in image galleries or sliders to display additional information when an image is hovered over or clicked on.
- Color Overlay: A color overlay involves placing a semi-transparent color layer on top of an element to change its appearance. This is commonly used for hover effects, dimming background images, or highlighting certain areas of a webpage.
- Modal Overlay: A modal overlay is a fullscreen or centered popup that appears on top of the webpage content to display additional information or functionality. Modal overlays are commonly used for displaying forms, notifications, or other important messages without navigating away from the current page.
- Video Overlay: A video overlay involves displaying a video on top of other content, often with transparency or blending effects. Video overlays can be used for video backgrounds, interactive elements, or as part of multimedia presentations.
- Text Overlay: A text overlay is when text is placed on top of an image or background, typically used for captions, labels, or annotations.
- UI Overlay: A UI overlay is used to provide visual feedback or interact with users without obstructing the main content. This could include tooltips, dropdown menus, or context menus that appear on top of specific elements when triggered.
How To Create an Overlay Effect | CSS
A CSS overlay is a technique used to create a semi-transparent layer on top of another element, typically to provide visual effects such as dimming the background or highlighting a particular area. This technique is commonly used in web development for modal dialogs, lightboxes, or image overlays.
Here's how you can create a CSS overlay:
HTML Structure
First, you'll need an HTML structure containing the content you want to overlay. For example, a <div> element with some content inside:
Overlay Structure
Next, create an overlay element. This element will be positioned on top of the content to create the overlay effect. It typically covers the entire viewport:
CSS Styling
Style the overlay to achieve the desired visual effect. You can adjust properties like background color, opacity, and position to customize the overlay. Here's an example CSS code:
css
In this example, rgba(0, 0, 0, 0.5) defines a semi-transparent black color for the overlay. The rgba() function allows specifying the color using red, green, and blue values along with an alpha value (opacity). Here, the alpha value of 0.5 makes the overlay 50% transparent.
Full Source:Techniques for Creating CSS Overlays
Using position and opacity
This is the most basic method. Create a separate element for your overlay and position it absolutely (position: absolute) on top of the content you want to cover. Then, set its opacity to a value between 0 (fully transparent) and 1 (fully opaque) to achieve the desired transparency level.
HTML :This HTML code contains two <div> elements: one for the content and another for the overlay. The CSS positions the overlay on top of the content using absolute positioning and sets its initial opacity to 0 to make it hidden. Adjustments have been made to ensure the overlay appears centered both horizontally and vertically and uses a white text color.
Using pseudo-elements
You can use pseudo-elements like ::after or ::before to create an overlay directly on the element itself. This approach is useful when you want the overlay to be tightly coupled with the element.
HTML :
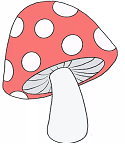
This HTML code contains a <div> element with an image inside. The CSS adds an overlay effect using the ::after pseudo-element. The overlay is initially hidden with an opacity of 0, but it becomes visible when hovering over the image due to the :hover selector. Adjust the background-color and opacity values as needed to achieve the desired overlay effect.
Using backdrop-filter
This property allows you to apply a blur filter to the underlying content, creating a subtle overlay effect. However, it has limited browser support.
CSS:Triggering the Overlay
inally, you may need JavaScript to trigger the overlay, such as when a user clicks a button or performs a certain action. You can use JavaScript to toggle the visibility of the overlay element.
Here's a simple example using JavaScript to toggle the overlay when a button is clicked:
With this setup, clicking the button will toggle the active class on the overlay, which can be used to control its visibility through CSS.
Full Source:Image hover effect
Create an overlay with additional information that appears when hovering over an image.

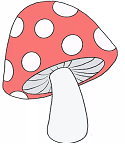
The .overlay class defines a fixed-position overlay covering the entire viewport with a blur effect applied using the backdrop-filter property. It is initially hidden with an opacity of 0. The .overlay.active class is used to make the overlay visible by changing its opacity to 1. JavaScript is included to toggle the active class on the overlay when a button is clicked. Adjust the backdrop-filter value and other styles as needed to achieve the desired appearance for the overlay.
Modal windows
Build pop-up windows that overlay the main content and require user interaction.

- The modal window is initially hidden (display: none) using the .modal class.
- The modal is displayed as a semi-transparent black overlay covering the entire viewport with a z-index to ensure it appears above other content.
- The actual modal content is positioned in the center of the viewport using absolute positioning and transform: translate(-50%, -50%).
- A close button (×) is positioned in the top-right corner of the modal content and has a click event listener to close the modal when clicked.
- Clicking the "Open Modal" button triggers the openModal() function, which adds the .active class to the modal, making it visible.
- Clicking the close button triggers the closeModal() function, which removes the .active class from the modal, hiding it again.
Conclusion
A CSS overlay is a technique used to create visual effects such as dimming the background or highlighting specific content by placing a semi-transparent layer on top of another element. This overlay is typically achieved using CSS properties like position, background-color, opacity, and z-index can be triggered through user interaction or JavaScript events. It's commonly used for modal dialogs, lightboxes, image overlays, and other UI enhancements in web development.