ByteArray from Stream c#
In C#, you can convert a byte[] (byte array) to a Stream using the MemoryStream class, which is a stream that uses a byte array as its backing store. Here's how you can do it:
Using MemoryStream
The MemoryStream class allows you to create a stream that reads from or writes to a byte array. To convert a byte[] to a MemoryStream, you can simply pass the byte array as an argument to the MemoryStream constructor.
In this example, we created a sample byte[] named byteArray, and then we created a MemoryStream named stream using that byte array.
Using MemoryStream with using statement
It is a good practice to use the using statement with MemoryStream to ensure proper disposal of resources, especially if you are working with large byte arrays or when the stream is no longer needed.
The using statement ensures that the MemoryStream is disposed of correctly, even if an exception is thrown within the block.
Converting byte[] to Stream in a method
If you want to create a method that converts a byte[] to a Stream, you can do it like this:
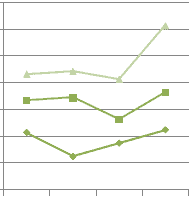
In this example, we created a method named ConvertByteArrayToStream, which takes a byte[] as input and returns a Stream using MemoryStream.
Remember to include the System.IO namespace at the beginning of your C# file when working with streams and MemoryStream.
Conclusion
Converting byte[] to Stream allows you to work with byte array data as if it were a stream, which can be useful for various scenarios, such as reading data from a byte array or passing data to methods that expect a stream as input.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#