String to byte Array
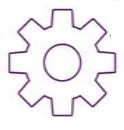
In C#, you can convert a string to a byte array using the Encoding class, which provides methods for encoding and decoding character data. The most commonly used encoding is UTF-8, but you can choose other encodings like UTF-16, ASCII, etc., depending on your requirements. Here's how you can convert a string to a byte array in C#:
Using UTF-8 encoding (default)
Using ASCII encoding
Using UTF-16 encoding
Remember that the byte array represents the encoded form of the string using the chosen encoding. If you need to convert the byte array back to the original string, you can use the corresponding Encoding class method, such as Encoding.UTF8.GetString(byteArray) for UTF-8 encoding.
Conclusion
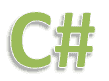
Keep in mind that different encodings have different byte representations for characters, so choose the appropriate encoding based on your specific use case and the data you are dealing with. UTF-8 is generally recommended for general use due to its compatibility with various characters and languages.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#