How to create word cloud using Python?
A word cloud is a visual representation of text data where the most frequently occurring words are displayed in a larger font size and are arranged in a way that reflects their relative importance. It is a popular technique for quickly identifying the most common words in a given text or group of texts, and is often used to analyze social media posts, customer reviews, news articles, and other types of textual data.
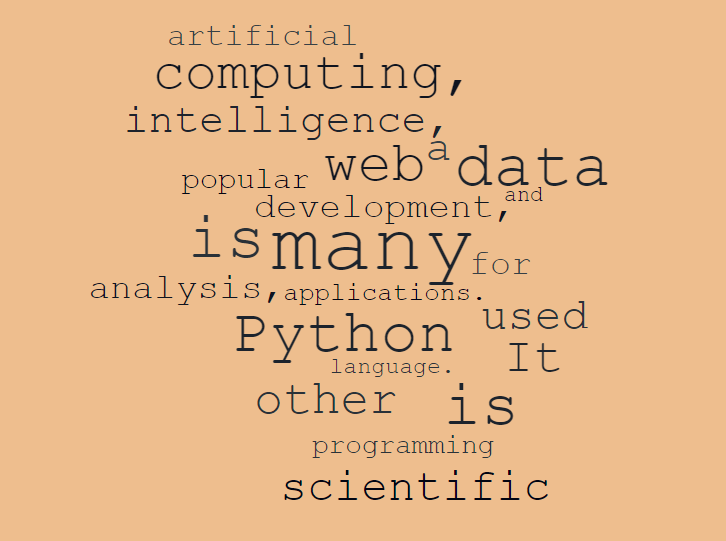
Word clouds are generated using software tools that automatically extract the most frequently occurring words from a given text, and then display them in a graphical format. The size and color of each word in the cloud are typically determined by its frequency, with more frequent words appearing larger and more prominent than less frequent words.
Word Cloud in Python
In Python, it is relatively easy to create word clouds using several popular libraries, including wordcloud, matplotlib, and numpy.
Installing Libraries
First, you need to install the required libraries. Open your command prompt or terminal and type the following commands:
These commands will install wordcloud, matplotlib, and numpy libraries.
Importing Libraries
After installation, you need to import the libraries in our Python script. Here's how you can import them:
Import WordCloud class from wordcloud library, pyplot from matplotlib, and numpy for some basic calculations.
Preparing the Text Data
Before generating the word cloud, you need to prepare the text data. You can either read the text data from a file or provide it as a string. For this tutorial, provide the text data as a string.
Creating the Word Cloud Object
Next, you need to create a WordCloud object. You can set various parameters for the object, such as the background color, the maximum number of words to display, the font size, and the width and height of the image. Here's how you can create the WordCloud object:
In the above code, set the width and height of the image to 800 pixels and the background_color to white. You can also set stopwords to an empty list, which means that you will not remove any common words from the text. Finally, set the min_font_size to 10.
Displaying the Word Cloud
Once you have created the WordCloud object, you can display it using the pyplot library. Here's how you can display the word cloud:
In the above code, set the figsize parameter to 8x8 inches, set the facecolor to None, and displayed the image using imshow() function. Also turned off the axis using axis("off") function and used tight_layout() to optimize the spacing between the words. Finally, displayed the image using show() function.
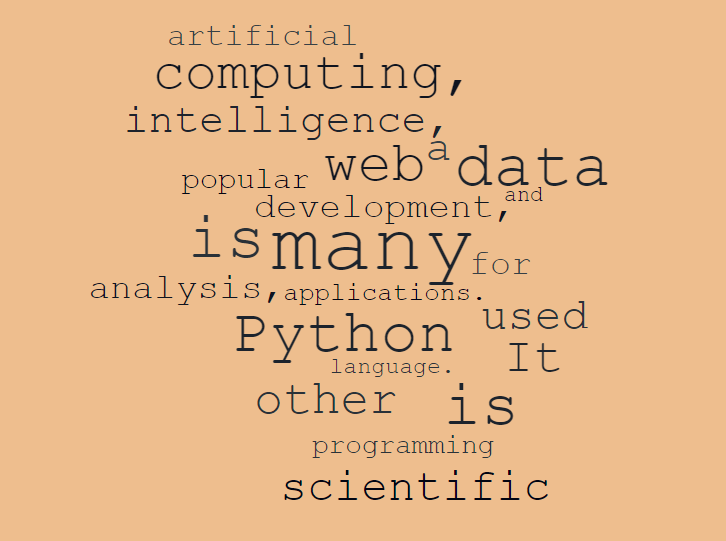
Saving the Word Cloud
You can also save the word cloud as an image file.
This code will save the word cloud as a PNG image file with the name wordcloud.png in the current working directory.
When to Use a Word Cloud
Word clouds can be used in a variety of situations where you want to visually represent text data in a way that highlights the most frequent words or topics. Here are a few examples of when a word cloud might be useful:
- Exploring social media sentiment: Word clouds can be used to identify the most commonly used words in social media posts related to a particular topic or brand. This can help businesses understand the sentiment of their customers and identify areas for improvement.
- Analyzing customer feedback: Word clouds can be used to analyze customer feedback from surveys or reviews, allowing businesses to quickly identify the most common issues or concerns that customers have.
- Summarizing news articles: Word clouds can be used to summarize the key themes and topics covered in news articles, making it easier to quickly understand the main points of the article.
- Identifying trends in research: Word clouds can be used to identify the most frequently occurring words in research papers or academic articles, helping researchers identify trends and areas of focus within a particular field.
Overall, word clouds can be a useful tool for quickly summarizing and visualizing large amounts of text data. However, it's important to use them in conjunction with other analytical techniques to ensure that the insights gleaned from them are accurate and meaningful.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python