Unindent does not match any outer indentation
In Python, indentation is used to indicate the grouping and hierarchy of statements within a code block. The standard indentation level in Python is four spaces, but tabs or any number of spaces can also be used.
When the indentation of a code block is incorrect or inconsistent, it can result in an IndentationError. One common type of IndentationError is the unindent does not match any outer indentation level error, which occurs when the indentation of a line of code does not match the indentation level of the surrounding block.
Mismatch of Indent size in code block
Following is an example of the Indentationerror:unindent does not match any outer indentation level error:
In the above example, the line print("How are you?") is indented with an extra four spaces compared to the previous line, print("Hello, world!"). This causes an IndentationError because the indentation of the second line does not match the indentation of the surrounding block.
To fix the error, you need to make sure that all the lines within the function have the same indentation level. Here's the corrected code:
In the above corrected code, both print statements have the same indentation level, which matches the indentation level of the surrounding function block. This code should run without any IndentationError.
Mismatch of Indent size outside of code block
Another example of the unindent does not match any outer indentation level error is shown below:
In the above example, the line print("x is not zero.") is indented with two spaces instead of the standard four spaces. This causes an IndentationError because the indentation level of the second line does not match the indentation level of the surrounding if block.
To fix the error, we need to ensure that all the lines within the if block have the same indentation level. Here's the corrected code:
In the above corrected code, both print statements have the same indentation level, which matches the indentation level of the surrounding if block. This code should run without any IndentationError.
Indentation in Python
Indentation is a unique feature of the Python programming language that is used to indicate the grouping and hierarchy of statements within a code block. Indentation is mandatory in Python, and it is used instead of traditional braces or curly brackets used in other programming languages to denote code blocks.
In Python, the standard indentation level is four spaces, but tabs or any number of spaces can be used as long as they are consistent within the same code block. However, mixing tabs and spaces within the same code block is not allowed and will result in an IndentationError.
It is important to maintain consistent indentation throughout the code to avoid IndentationError and ensure that the code is easy to read and understand. The choice of the number of spaces or tabs to use for indentation is a matter of personal preference, but it is recommended to stick to the standard of four spaces.
How to check for a mix of tabs and spaces
Mixing tabs and spaces in indentation can cause IndentationError and make the code difficult to read and understand. Here's how to check for a mix of tabs and spaces in Python indentation:
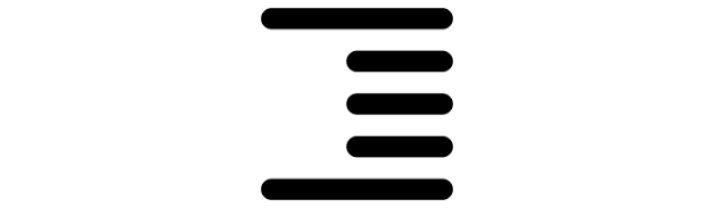
Manual Inspection:
The easiest way to check for a mix of tabs and spaces in Python indentation is to visually inspect the code. In most text editors, tabs are represented by a wider whitespace than spaces, making it easier to spot inconsistencies in indentation.
Use Python's "Tabnanny" tool:
In Python, indentation is an essential aspect of the syntax and is used to indicate the grouping and hierarchy of statements within a code block. Improper indentation can cause IndentationError and make the code difficult to read and understand. To avoid such errors, Python provides a tool called tabnanny, which can be used to track and report indentation errors in a Python script.
Tabnanny is a built-in tool in Python that scans a Python script for indentation errors caused by mixing tabs and spaces or inconsistent indentation levels. The tabnanny module can be run from the command line or within a Python script to check for indentation errors.
Following is an example of how to use tabnanny to check a Python script for indentation errors:
In the above command, tabnanny is invoked using the -m option, followed by the name of the Python script to be checked (script.py in this case). Tabnanny scans the script and reports any indentation errors that it finds.
If tabnanny detects an indentation error, it will display an error message that indicates the location and nature of the error. The error message will include the line number, the character position within the line, and a brief description of the error.
Following is an example of an IndentationError that is detected by tabnanny:
This error message indicates that there is an inconsistency in the use of tabs and spaces within the indentation of the Python script, and the error is located in line 11 of the tabnanny.py file.
Use Python's "Whitespace" module:
Python also has a built-in module called whitespace that can be used to check for a mix of tabs and spaces in Python indentation.
Replace myfile.py with the name of the Python file you want to check. If the whitespace module finds any inconsistencies in indentation, it will raise an exception with a message indicating the line number and location of the error.
Show Whitespace:
Another way to check for a mix of tabs and spaces in Python indentation is to enable the "Show Whitespace" feature in your text editor. This feature displays tabs and spaces as special characters in the code editor, making it easy to spot inconsistencies in indentation.
Conclusion:
Resolving Python indentation errors can be done easily using the built-in tools of popular IDEs. By following these steps, Python programmers can quickly fix indentation errors and ensure their code is properly indented, making it easier to read and understand.