Enum Flags Explained
Enum
An Enumeration (Enum) type offers an effective and optimized approach for defining a collection of named integral constants that can be assigned to variables.
Enum Flags Attribute
![What Does the [Flags] Attribute Really Do c# asp.net vb.net](img/root.png)
The concept of Enum Flags is designed to enable an enumeration variable to hold multiple values simultaneously. It is recommended to utilize Enum Flags when the enumeration represents a collection of flags rather than a singular value. These flag-based enumeration collections are commonly manipulated using bitwise operators. To create a bit flags enum, the System.FlagsAttribute attribute is applied, and the values are defined appropriately to facilitate bitwise operations such as AND, OR, NOT, and XOR on them.
C#You can use like this:
In conditions:
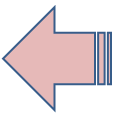
To properly implement an enum as a flag, you need to have the values increasing by a power of two (2). You should define enumeration constants in powers of two, that is, 1, 2, 4, 8, and so on. It is important, because it allows us to do bit-wise operations on the number. The values themselves can easily be calculated by raising 2 to the power of a zero-based sequence of numbers like the following:

yields this (yes, 2 to the zero power is one):
1, 2, 4, 8, 16, 32, 64
This means the individual flags in combined enumeration constants do not overlap.
Unlike the old enums, the actual values in a flags enumerator must be in sequence for it to work correctly. This does not refer to a base 10 sequence, but instead to a base 2 sequence. Hence the Character enumeration can now act in Bitwise Operators. The values of the Enum will look like the following:
What it is explain that the integer value expressed as base 2 must be as shown (for four values in the above example): 00001 00010 00100 01000 . Expressed in base 10, this is 1, 2, 4, 8.
Difference :You can see the difference lies in the Enum.ToString() method. If your enum has the [Flags] attribute set then the ToString() will return a comma seperated values list of bitwise enum values. If there's no [Flags] attribute ToString() will return a number for every bitwise value.
C# Source CodeOutput : 5 - with flag : Low, High
VB.Net Source CodeOutput : 5 - with flag : Low, High
NOTE:
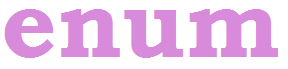
While it is true that C# allows developers to perform bitwise operations on enumerations without explicitly applying the FlagsAttribute, it is important to note that Visual Basic (VB.Net) does not provide this flexibility. Therefore, if you are exposing your types to other programming languages or working in a multi-language environment, it is beneficial to mark the enumeration with the FlagsAttribute. This practice not only ensures compatibility across languages but also conveys a clear intention that the members of the enumeration are intended to be used together as a collection of flags.
Conclusion
By applying the FlagsAttribute, you establish a standard and promote better understanding and usage of the enumeration within the development ecosystem.