How to Refresh/Reload a Page with jQuery/JS
The JavaScript reload() method is utilized to refresh the current webpage or navigate to another URL by using the location object. It allows for reloading the current resource and obtaining the URL of the present page. Additionally, it can be used to redirect the browser to a different page for navigation purposes.
location.reload()
The location.reload() method is indeed a standard JavaScript method used to reload the current webpage. jQuery is not required for this functionality. The method accepts an optional parameter, which by default is false, indicating that the page may be reloaded from the browser's cache. If you want to force the browser to retrieve the document from the web server (useful when the content changes dynamically), you can pass true as the argument.
Moreover, location.reload() is compatible with all major browsers, including IE, Chrome, Firefox, Safari, and Opera. It's a simple and widely used method for refreshing or reloading a web page in JavaScript.
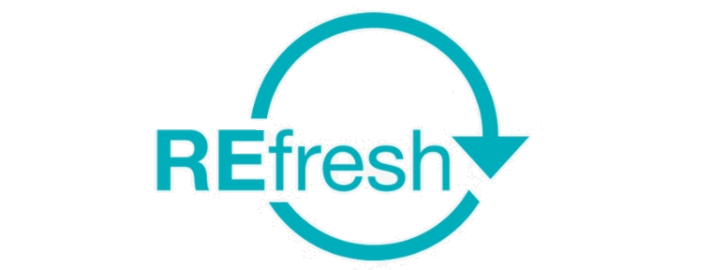
JavaScript window.location object can be used:
- Get current page address (URL)
- Redirect the browser to another page
- Reload/refresh the same page

In jQuery/JavaScript, there are lots of ways reload/refresh a page:
history.go(0);
The History interface indeed provides a means to manage the browser's session history, which includes the pages visited in the current tab or frame.
The history.go() method allows you to navigate the browser's history by a specific number of steps. Passing a parameter of '0' to the method reloads the current page, effectively refreshing it without changing the history state. This is a useful technique for reloading a page without affecting the browsing history.
If the current page was loaded by a POST request , you may want to use
There are multiple ways to Refresh/Reload a page with jQuery/JavaScript, some are:
- location.href = location.href
- location.replace(location.pathname)
- window.location = window.location
- window.self.window.self.window.window.location = window.location
Refresh a page after certain delay jQuery
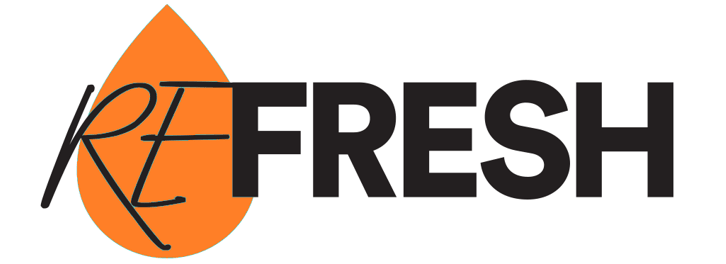
The setTimeout() method is commonly used to execute code after a specified period of time. It takes two parameters: the first is the function you want to execute, and the second is the delay in milliseconds before the function is executed.
In your explanation, you rightly mentioned that the delay value is provided in milliseconds. If you want to delay the execution in seconds, you need to multiply the number of seconds by 1000 to convert it to milliseconds. This is a useful approach for creating time-based actions, like automatically refreshing a page after a certain interval.
The reload may be blocked and a SECURITY_ERROR DOMException thrown. This happens if the origin of the script calling location.reload() differs from the origin of the page that owns the Location object.
Conclusion
To reload a page using jQuery, you can utilize the location.reload() method, which is a standard JavaScript feature and does not require jQuery. It refreshes the current webpage, optionally fetching the document from the server if the argument is set to true. jQuery is not necessary for this operation.