jQuery DOM Manipulation
What is DOM?
The Document Object Model (DOM) is a conceptual model depicting the structural composition of a "document." Serving as a language and platform-agnostic interface, it enables dynamic interaction and modification of a document's content, structure, and styling through scripts and programs.
DOM Structure
The Document Object Model (DOM) offers an organized portrayal of a document's structure, furnishing a systematic means by which programs can access and modify the document's arrangement, style, and content. At the core of this tree-like structure lies the Document Object, encompassing various subordinate child objects. Additionally, the document object comprises several properties that provide insights into the overall state of the document. For instance, the property document.title corresponds to the title of the current page, a descriptor defined by the HTML < title > tag.
HTML Document
The Document Object Model (DOM) ensures a consistent portrayal of an HTML document by depicting the entire document as a hierarchical tree structure. As a web page is loaded within a browser, a corresponding Document Object Model is constructed to mirror its structure. Every individual element present within the document corresponds to a distinct entity within the DOM, encouraging a one-to-one correspondence between the two representations.
Sample HTML Page:
HTML Dom Structure
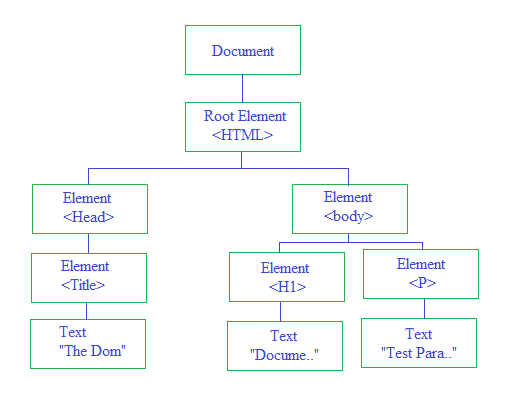
The Document Object Model (DOM) is presently divided into two components: DOM Core and DOM HTML. DOM Core forms the foundation for both XML and HTML documents, and all DOM implementations are required to support the fundamental interfaces outlined in the Core specification. For XML implementations, supporting the "extended" interfaces in the Core specification is also mandatory. The Level 1 DOM HTML specification introduces supplementary functionality tailored specifically for HTML documents.
DOM and JavaScript
JavaScript, being an interactive language, is best comprehended through practical engagement. The Document Object Model (DOM) complements this by providing a structural framework for web pages, HTML documents, XML documents, and their constituent elements. While the DOM itself isn't a programming language, it is indispensable to JavaScript as it furnishes the necessary structure for JavaScript to interact with web content, thereby establishing JavaScript as a client-side scripting language that seamlessly interfaces with the DOM in web browsers.
DOM and jQuery
jQuery is a JavaScript library designed for dynamic modification of web documents. Equipped with a plethora of methods, jQuery simplifies DOM traversal, the process of moving through a web page's structure. The DOM, or document object model, serves as the bridge that facilitates JavaScript's interaction with web page content. Whether using JavaScript or jQuery, when we inspect or manipulate elements on a web page, we are effectively engaging with the DOM. In upcoming chapters, we'll investigate into navigating the DOM hierarchy, manipulating parent-child relationships, and dynamically altering page structure using jQuery's capabilities.