setInterval Vs setTimeout in JavaScript
In JavaScript, activities that are planned to occur at specific time intervals in the future are commonly referred to as scheduling. JavaScript provides functions and methods that allow developers to execute code at predetermined times or intervals. They are:
- setTimeout(function, milliseconds)
- setInterval(function, milliseconds)
Both the functions allow you to execute a piece of JavaScript code/function at a certain point in the future.
setTimeout(function, milliseconds)
JavaScript setTimeout() function is utilized to execute a specified JavaScript statement after a defined time interval, denoted as 'X'. This method facilitates time-based code execution, ensuring that the script is executed once when the specified interval elapses. For repeated execution, developers can opt to loop the script by nesting the setTimeout() object inside the function it calls to run. However, if the looped execution requires termination, the clearTimeout() method can be employed to halt the ongoing interval-based firing.
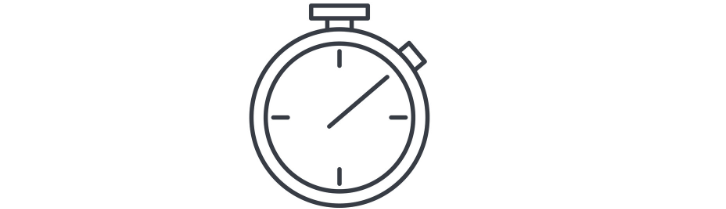
This careful management of the setTimeout() function enables precise control over the timing and repetition of code execution, optimizing the performance and behavior of time-sensitive operations within JavaScript applications.
If you want something to happen one time after a specified period of time, then use setTimeout(). That is because it only executes one time when the specified interval is reached.
setInterval(function, milliseconds)
JavaScript setInterval() function is utilized to execute a specified JavaScript statement at regular intervals, denoted as 'X'. This method offers a time-based code execution approach, allowing for the repetitive execution of a designated script whenever the specified interval is reached. Unlike setTimeout(), setInterval() inherently possesses the ability to loop the script without the need for nesting it into its callback function. This looping behavior occurs by default, making it convenient for continuous execution. To terminate the ongoing interval-based firing, developers can employ the clearInterval() method. By skillfully managing the setInterval() function and clearInterval() method, programmers can ensure precise control over the periodic execution of code, optimizing the performance and behavior of time-driven operations within JavaScript applications.
If you want to loop code for animations or on a clock tick, then use setInterval().
Accuray (setTimeout Vs. setInterval)
Please note that all scheduling methods do not guarantee the exact delay. For example, the in-browser timer may slow down for a lot of reasons:
- The CPU is overloaded.
- The browser tab is in the background mode.
- The laptop is on battery.
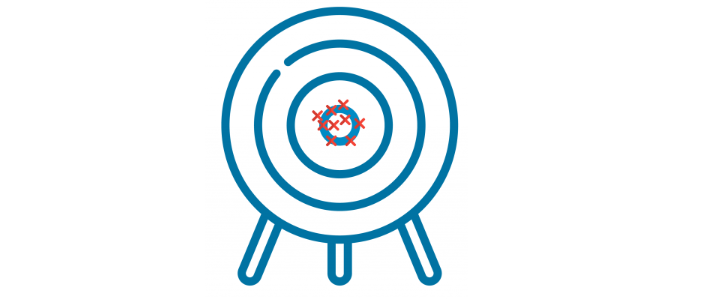
Both setTimeout and setInterval serve the purpose of executing code at specific time intervals in JavaScript. However, there are subtle differences in their behavior that can impact accuracy.
With setTimeout, the function is executed after the specified delay, and then a new timeout is set for the next execution. This means there might be a slight delay between each execution, resulting in a cumulative error over time, leading to a less accurate timing compared to the desired interval.
On the other hand, setInterval attempts to execute the function repeatedly at the specified interval. However, due to JavaScript's single-threaded nature, if other parts of the script are running, the interval execution might be delayed to ensure smooth execution of the entire script. This can result in minor variations in the interval timings, which can accumulate over time.
Moreover, the minimal timer resolution (the minimal delay) can vary based on the browser and OS-level performance settings. In some cases, this minimum delay can be around 300ms or even 1000ms, which further impacts the accuracy of setTimeout and setInterval.
Conclusion
In practical scenarios, for most applications, these timing discrepancies are negligible and won't significantly affect the performance of the code. However, if precise timing is essential, developers can use techniques like performance monitoring or employing the Date object to accurately measure the time elapsed between each execution.
- Difference between using "let" and "var" in JavaScript
- How to Use Array forEach() in JavaScript
- How to Remove Duplicate Values from a JavaScript Array?
- How To Get a Timestamp in JavaScript
- Function Declarations vs. Function Expressions in JavaScript
- How to append values to an array in JavaScript?
- How To Remove a Property from a JavaScript Object
- Which equals operator (== vs ===) should be used in JavaScript comparisons?
- How to Capitalize the First Letter of Each Word in JavaScript
- Get the size of the screen/browser window | JavaScript/jQuery
- Can't set headers after they are sent to the client
- Blocked by CORS policy: No 'Access-Control-Allow-Origin'