Difference between var and let in JavaScript
The var keyword was introduced with JavaScript while the let keyword was added in ES6 (ES 2015) version of JavaScript.
Scoping rules
Variables declared using the "var" keyword are confined to the immediate function body, constituting what is known as function scope. On the other hand, variables defined with the "let" keyword are limited to the immediate enclosing block, typically identified by {....}, thus embodying block scope.
Understanding the distinction between these two types of variable scopes is crucial for proficiently managing data and ensuring the appropriate visibility and accessibility of variables within different sections of code. By employing the appropriate scope for variables, developers can enhance code organization, reduce potential conflicts, and support a more structured and maintainable codebase.
Using varIn the above example, you can see a variable defined using a var statement is known throughout the function it is defined in, from the start of the function. Also, a variable defined using a let statement is only known in the block it is defined in, from the moment it is defined onward.
Re-declarations
Variables declared using the "var" keyword have the flexibility of being both re-declared and updated within the same scope. This means that you can use the "var" keyword to define a variable multiple times in the same block of code, and also modify its value during the execution of the program.
On the other hand, variables declared using the "let" keyword offer a different behavior. While they can be updated with new values, they cannot be re-declared within the same scope. This means that once you declare a variable with "let" in a specific block, you cannot use the "let" keyword to declare it again in that same block.
This distinction between "var" and "let" adds an extra layer of control and predictability to the way variables are managed in JavaScript code. Utilizing "let" for variables that should not be re-declared in the same scope can help prevent accidental variable shadowing and improve code clarity and maintainability.
Using var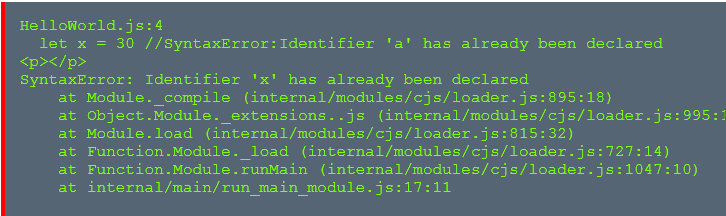
JavaScript hoisting
Hoisting in JavaScript refers to the automatic process of moving all variable declarations to the top of their respective scopes, be it global or functional. Consequently, variables can be utilized before being formally declared. Nevertheless, it is essential to understand that variables declared with the "let" keyword are hoisted to the top of their block but remain uninitialized until explicitly declared. Thus, while the code block recognizes the existence of the "let" variable, it cannot be utilized until its declaration statement is reached. Attempting to use a "let" variable before its declaration will lead to a ReferenceError, indicating that the variable is not yet defined in the current scope. It is prudent to adhere to proper variable declaration practices to ensure code correctness and avoid potential issues arising from hoisting behavior.
Using var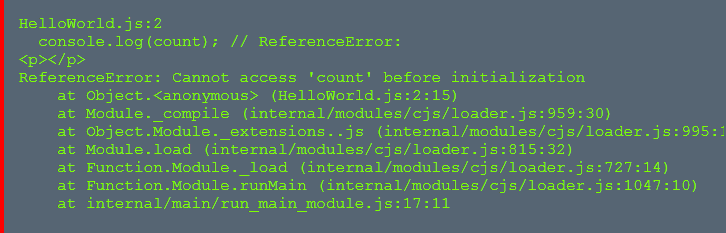
Pros and Cons (var Vs. let)
Opting for block scoping is considered the standard and more legible approach, as it enhances the readability and maintainability of code, making debugging a smoother process. Block scoping offers the advantage of clearly delineating the exact scope of a variable within a code block, thereby aiding developers in easily discerning its intended usage and preventing inadvertent errors stemming from scoping mishaps. Conversely, function scoping can be less straightforward and obscure, increasing the likelihood of introducing bugs due to scoping errors. By adopting block scoping, developers can support a more structured and error-resistant codebase, promoting code clarity and overall software quality.
When to use var or let?
Due to their limited scope, "let" variables are typically employed when the variables have constrained and specific usage, such as within the context of for loops, while loops, or if conditions. The use of "let" ensures that the variables are confined to the appropriate blocks, preventing unintentional conflicts or unintended access outside of those blocks. This promotes better code organization and reduces the risk of unexpected behavior.
On the other hand, "var" variables are utilized when the value of the variable is meant to be less prone to changes and requires global accessibility. "var" declarations are hoisted to the top of their scope, and their reach extends beyond blocks, making them suitable for cases where the variable's value needs to be accessible throughout the entire function or even globally. However, this broader scope can sometimes lead to unintended consequences and potential conflicts, hence the recommendation to use "let" and "const" for more localized and controlled variable declarations.
Conclusion
Making careful decisions about which type of variable to use based on their intended scope and usage, developers can improve code predictability, maintainability, and minimize potential issues related to variable scoping.
- How to Use Array forEach() in JavaScript
- How to Remove Duplicate Values from a JavaScript Array?
- How To Get a Timestamp in JavaScript
- Function Declarations vs. Function Expressions in JavaScript
- How to append values to an array in JavaScript?
- How To Remove a Property from a JavaScript Object
- Which equals operator (== vs ===) should be used in JavaScript comparisons?
- How to Capitalize the First Letter of Each Word in JavaScript
- Get the size of the screen/browser window | JavaScript/jQuery
- Difference between setInterval and setTimeout in JavaScript
- Can't set headers after they are sent to the client
- Blocked by CORS policy: No 'Access-Control-Allow-Origin'