Remove specific properties from Array objects | JavaScript
Managing data often involves the need to remove specific elements from JavaScript arrays, and while there is no single built-in 'remove' method, there are various techniques available to achieve this task effectively. Removing properties or fields from an array of objects is particularly crucial when dealing with sensitive tabular data.
One common approach to remove items from an array of objects is to use the filter() method along with a callback function that specifies the condition for removal. The filter() method creates a new array with elements that pass the given condition, effectively omitting the unwanted items from the original array.
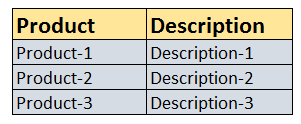
Using array.forEach()
Method - 2
Using Array.prototype.map()
The map() method is used to create a new array with the results of calling a provided function on each element of the original array. It iterates through each element in the array, invokes the specified callback function on each element, and constructs a new array containing the results returned by the callback function.
Conclusion
Using the map() method enables concise and functional programming approaches, making it a valuable tool for various array manipulation tasks in JavaScript.
- Difference between using "let" and "var" in JavaScript
- How to Use Array forEach() in JavaScript
- How to Remove Duplicate Values from a JavaScript Array?
- How To Get a Timestamp in JavaScript
- Function Declarations vs. Function Expressions in JavaScript
- How to append values to an array in JavaScript?
- Which equals operator (== vs ===) should be used in JavaScript comparisons?
- How to Capitalize the First Letter of Each Word in JavaScript
- Get the size of the screen/browser window | JavaScript/jQuery
- Difference between setInterval and setTimeout in JavaScript
- Can't set headers after they are sent to the client
- Blocked by CORS policy: No 'Access-Control-Allow-Origin'