jQuery Dimensions
Within the jQuery library, a range of methods are at your disposal to manipulate the dimensions of DOM elements, encompassing attributes such as height and width. When crafting reusable plugins, there arises a frequent need to establish or animate an element's dimensions, accounting for its padding, border, and margin. This becomes particularly significant in plugins designed to accommodate bespoke styling preferences, ensuring a comprehensive and accurate representation of the element's visual layout.
jQuery provides six functions that we can use to get the height and width of an element.
- width()
- height()
- innerWidth()
- innerHeight()
- outerWidth()
- outerHeight()
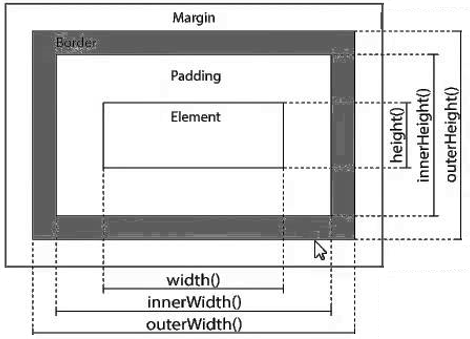
jQuery width() and height() Methods
The jQuery width() and height() methods serve to retrieve or establish the width and height of an element, respectively. However, it's important to note that these dimensions solely pertain to the element's content area and exclude any padding, border, and margin that may be present.
The above code will return the width and height of the boxDiv element.
innerWidth() and innerHeight() Methods
jQuery innerWidth() and innerHeight() methods provide values for the inner width and inner height of an element, encompassing its padding. These dimensions offer a holistic view of the element's content area along with its associated padding, without accounting for borders or margins.
The above code will return the Inner width and Inner height of the boxDiv element.
- InnerHeight = height + padding on both side = 100 + 20 + 20 = 140
- InnerWidth = width + padding on both side = 200 + 20 + 20 = 240
outerWidth() and outerHeight() Methods
jQuery outerWidth() and outerHeight() methods furnish measurements for the outer width and outer height of an element, encompassing both padding and border. It's important to note that these dimensions account for the padding and border, while excluding the margin of the element.
The above code will return the Outer width and Outer height of the boxDiv element.
- OuterHeight = height + padding + border = 100 + 20 + 20 + 2 + 2= 144
- InnerWidth = width + padding + border = 200 + 20 + 20 +2 +2 = 244
outerWidth(true) and outerHeight(true)
jQuery outerWidth(true) and outerHeight(true) methods provide the values for the outer width and outer height of an element, encompassing padding, border, and margin. These dimensions offer a comprehensive representation of the entire element, including all visual aspects that contribute to its layout and spacing.
- OuterHeight(true) = height + padding + border + margin = 100 + 20 + 20 + 2 + 2 + 5 + 5= 154
- InnerWidth(true) = width + padding + border + margin = 200 + 20 + 20 +2 +2 +5 +5 = 254

Width:
Inner Height:
Outer Width:
Outer Width(true)
Conclusion
jQuery offers a suite of methods to manage the dimensions of DOM elements. The width() and height() methods retrieve or set content dimensions, while innerWidth() and innerHeight() include padding. outerWidth() and outerHeight() incorporate padding and border, while outerWidth(true) and outerHeight(true) encompass padding, border, and margin for a comprehensive measurement.