JavaScript if/else Statement
JavaScript's If...Else statements are fundamental constructs that enable developers to create conditional logic, allowing the execution of different code blocks based on specified conditions. Here's a comprehensive explanation of If...Else statements with examples:
If Statement
The basic form starts with the if keyword, followed by a condition enclosed in parentheses. If the condition evaluates to true, the code block within the curly braces {} is executed.
If...Else Statement
By extending the if statement with an else clause, you can provide an alternative code block to be executed when the condition evaluates to false.
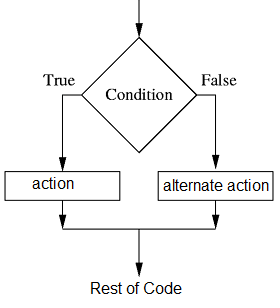
If...Else If...Else Statement
To handle multiple conditions, you can use else if clauses between the initial if and the final else.
Nested If...Else Statements
If...Else statements can be nested, allowing for more intricate conditional flows.
Ternary Operator (Conditional Operator)
For concise conditional assignments, the ternary operator can be used as a shorthand.
Conclusion
If...Else statements form the backbone of decision-making in JavaScript, allowing developers to control the flow of their programs based on various conditions, ultimately enhancing the logic and interactivity of their applications.