Parse XML - XmlDocument
The XmlDocument Class serves as a representation of an XML document, providing comprehensive capabilities for handling XML data. When using XmlDocument, the entire XML content is read into memory, allowing developers to efficiently navigate both backward and forward within the document. Additionally, it facilitates the implementation of XPath technology, enabling powerful querying functionalities for the XML document.
Upon reading the XML content into memory, XmlDocument provides a hierarchical representation of the XML structure, organizing elements and attributes in a tree-like format. This enables developers to access and manipulate the XML data in a structured manner, making it convenient to perform various operations, such as creating, modifying, or deleting elements and attributes.
XML Parser in C# and VB.Net
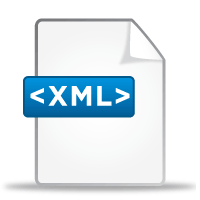
The XPath technology integration within XmlDocument further empowers developers to execute complex queries on the XML document, enabling them to extract specific data efficiently. XPath is a powerful query language for XML that allows for the selection and navigation of nodes within the XML document based on specific criteria, enhancing the flexibility and precision of data retrieval.
Using XPath with the XmlDocument class
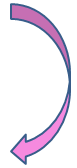
The XmlNodeList Class serves as a structured, ordered collection of nodes in XML data. When seeking specific nodes within an XML file, developers can utilize XPath expressions, a powerful query language for XML. With the XmlNode.SelectNodes method, one can easily retrieve a list of nodes that match the specified XPath string.
Using the XmlNodeList Class, developers can efficiently manage and access multiple nodes within the XML data. The class preserves the order of nodes, providing a convenient way to iterate through the collection and perform various operations on each node.