File.Exists Method
The File Class is a fundamental component of the .NET Framework that offers a collection of static methods to handle various file-related operations efficiently. It encompasses functionalities such as file creation, copying, deletion, moving, and opening, enabling developers to manage individual files with ease and precision.
Utilizing the File Class, developers can create new files, duplicate existing files, delete unwanted files, and move files to different locations seamlessly. Additionally, the class facilitates file opening operations, allowing for seamless interaction with file contents.
File.Exists Method
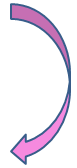
A particularly useful method provided by the File Class is File.Exists(), which serves as a valuable utility for checking the existence of a specific file. This method allows developers to verify the presence of a file before proceeding with further operations, ensuring robustness and error handling in file-related tasks.
Syntax
The File.Exists method is designed to return a Boolean value, offering a simple yet effective means of determining the existence of a file. When invoked, File.Exists will evaluate the specified path and assess whether the calling process possesses the necessary permissions to access the file and if the path indeed corresponds to an existing file. If these conditions are met, the method will return true; otherwise, it will return false. Furthermore, if an invalid path is provided to the File.Exists method, it will automatically return false, as it cannot correspond to an existing file.
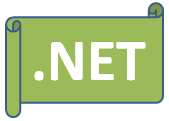
Due to its straightforward nature, File.Exists is ideally suited for use with the conditional operator (?), which allows developers to succinctly incorporate conditional logic into their code. By employing this operator in conjunction with File.Exists, developers can efficiently handle file-related tasks based on the existence of specific files, enhancing code readability and maintainability.
Search File using wild card
You can use wild card characters to check a file exists in a folder.
How to check if a file exists in a folder?
Source Code | C#
Source Code | Vb.Net
You can use searchoption enumeration to specifies whether to look in the current directory, or the current directory and all subdirectories.
SearchOption.AllDirectories
SearchOption.AllDirectories includes the current directory and all its subdirectories in a search operation.
SearchOption.TopDirectoryOnly
SearchOption.TopDirectoryOnly includes only the current directory in a search operation.
.Net Framework 4
If you are working with Microsoft .Net Framework 4 or a newer version, an optimal approach for file enumeration is to utilize the Directory.EnumerateFiles method. This method proves to be considerably more efficient than its counterpart, Directory.GetFiles, as it allows you to circumvent the need to iterate through the entire file list.
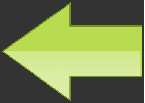
By using Directory.EnumerateFiles, you gain the advantage of lazy evaluation, which means the files are fetched and processed one by one as needed, as opposed to retrieving the entire file list upfront. This leads to enhanced performance and reduced memory overhead, especially when dealing with large directories containing numerous files. Thus, Directory.EnumerateFiles proves to be a superior choice for file enumeration in scenarios where efficiency and resource management are of vital importance.
Check File exist in Network Path
Conclusion
The File Class offers a set of static methods that streamline the management of individual files, making it a crucial tool for handling file operations in .NET applications. By providing a comprehensive suite of functionalities and utilities, the File Class enhances the efficiency and reliability of file manipulation tasks, ensuring smoother and more robust file handling within software applications.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#