Capitalize first letter of a string | JavaScript
There are number of ways to to capitalize a string to make the first character uppercase.
In the above code, uppercases the first character and slices the string to returns it starting from the second character.
example
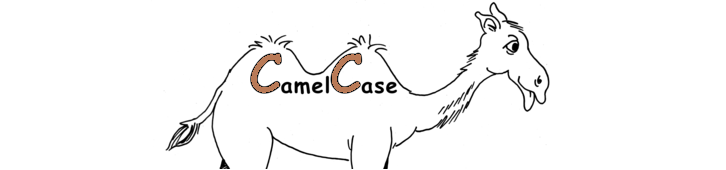
Using string.charAt()
Using string.replace()
Capitalize the first letter of each word
You can easily convert the first letter of each word of a string into uppercase by splitting the sentence into words using the split() method and applying charAt(0).toUpperCase() on each word.
JavaScript charAt()
JavaScript charAt() method returns the character at a specified index (position) in a string. The index of the first character is 0, the second 1, third 2 etc. The index of the last character is string length - 1
JavaScript toUpperCase()
JavaScript toUpperCase() method converts a string object into a new string consisting of the contents of the first string, with all alphabetic characters converted to be upper-case.
JavaScript slice()
The JavaScript array slice() method extracts the part of the given array and returns it.
Make first letter of a string uppercase in CSS
It's always better to handle these kinds of stuff using CSS first, in general, if you can solve something using CSS, go for that first, then try JavaScript to solve your problems, so in this case try using :first-letter :first-letter in CSS and apply text-transform:capitalize;
Conclusion
These methods provide different ways to capitalize the first letter of a string in JavaScript, catering to various preferences and coding styles. Choose the method that best suits your needs and coding context.
- Difference between using "let" and "var" in JavaScript
- How to Use Array forEach() in JavaScript
- How to Remove Duplicate Values from a JavaScript Array?
- How To Get a Timestamp in JavaScript
- Function Declarations vs. Function Expressions in JavaScript
- How to append values to an array in JavaScript?
- How To Remove a Property from a JavaScript Object
- Which equals operator (== vs ===) should be used in JavaScript comparisons?
- Get the size of the screen/browser window | JavaScript/jQuery
- Difference between setInterval and setTimeout in JavaScript
- Can't set headers after they are sent to the client
- Blocked by CORS policy: No 'Access-Control-Allow-Origin'