Blocked by CORS policy: No 'Access-Control-Allow-Origin'
Access to fetch at '' from origin '' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource is an error message that appears in the browser console when a web page is attempting to make an XMLHttpRequest (XHR) request to a resource hosted on a different domain or origin, and the server hosting the resource does not explicitly allow cross-origin requests from the domain of the web page making the request.
Cross-Origin Resource Sharing
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers that restricts web pages from accessing resources on a different domain, to prevent unauthorized access to sensitive data. If the server hosting the resource does not include the Access-Control-Allow-Origin header in its response, the browser will block the request and display the error message "No 'Access-Control-Allow-Origin' header is present on the requested resource".
How to fix "Blocked by CORS policy:.."
In order to resolve this error, the server hosting the resource needs to include the Access-Control-Allow-Origin header in its response, which specifies which domains are allowed to access the resource. This header tells the browser that the requested resource is intended for cross-origin access and which origins are allowed to access it.
Following are the possible ways to fix the "No 'Access-Control-Allow-Origin' header is present on the requested resource" error, along with detailed explanations and examples:
Check the server response
Use your browser's developer tools (e.g., Chrome DevTools) to check the server response for the resource you are trying to access. Look for the presence of the Access-Control-Allow-Origin header in the response. If it's not there, proceed to the next step.
Modify the server-side code:
If you have control over the server-side code, you can modify it to include the "Access-Control-Allow-Origin" header in the server response. The header value should either be set to the domain of the web page making the request, or to "*" to allow any origin to access the resource.
Following is an example of how to include the "Access-Control-Allow-Origin" header in a PHP script:
In the above example, the header value is set to "https://example.com", which means that only web pages hosted on that domain will be allowed to access the resource. If you want to allow any origin to access the resource, you can set the header value to "*":
Use a JSONP callback:
JSONP (JSON with Padding) is a technique that allows web pages to make cross-origin requests by using a script tag instead of an XHR request. The server responds with a JavaScript function call wrapped around the requested data, which the web page can then execute as a callback function.
Following is an example of how to use JSONP to request data from a server:
In the above example, the web page creates a new script tag with a src attribute pointing to the server endpoint that returns the data. The endpoint includes a query parameter named "callback" with the value set to the name of the callback function ("handleData" in this case). The server responds with a JavaScript function call that wraps the requested data, which the web page can then execute as the callback function.
JSONP is a simple and effective way to work around the same-origin policy, but it has some limitations, such as the inability to use POST requests or access response headers.
Use CORS headers:
CORS (Cross-Origin Resource Sharing) is a mechanism that allows web pages to make cross-origin requests, while still enforcing the same-origin policy to protect user data. When a web page makes a cross-origin request using XHR (XMLHttpRequest), the browser automatically sends an "Origin" header with the request, indicating the domain of the web page making the request. The server hosting the resource can then respond with a "Access-Control-Allow-Origin" header, indicating which domains are allowed to access the requested resource.
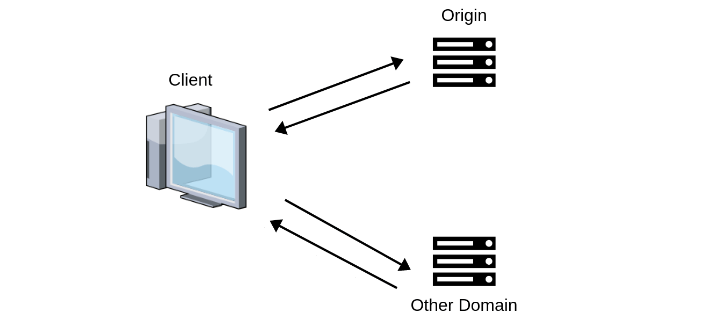
To fix the "No 'Access-Control-Allow-Origin' header is present on the requested resource" error using CORS headers, you need to modify the server-side code to include the "Access-Control-Allow-Origin" header in the server response. The header value should be set to either the domain of the web page making the request, or to "*" to allow any origin to access the resource.
Following is an example of how to use CORS headers to allow access to a resource from a different domain in a Flask web application:
Install the Flask-CORS extension:
Import the extension and add it to the Flask app:
Modify the route function to return the "Access-Control-Allow-Origin" header:
In the above example, the Flask app includes the Flask-CORS extension and uses it to enable CORS for all routes. The "get_data" route function returns a JSON response with the "Access-Control-Allow-Origin" header set to "", allowing any origin to access the resource. If you want to restrict access to specific domains, you can replace "" with the domain(s) you want to allow.
With these changes, the server should now include the "Access-Control-Allow-Origin" header in the response, allowing the web page to access the resource without triggering the "No 'Access-Control-Allow-Origin' header is present on the requested resource" error.
Use a reverse proxy:
If you don't have control over the server-side code, you can use a reverse proxy to add the "Access-Control-Allow-Origin" header to the server response. A reverse proxy is a server that sits between the client and the server hosting the resource, and can modify the headers of the server response before sending it back to the client.
Following is an example of how to use a reverse proxy to add the "Access-Control-Allow-Origin" header to a server response:
In the above example, the reverse proxy is configured to forward requests to "https://api.example.com/", and to add the "Access-Control-Allow-Origin" header to the server response with a value of "*", allowing any origin to access the resource.
Use a browser extension:
If you are only accessing the resource for testing purposes, you can use a browser extension that allows cross-origin requests. It's important to note that using a browser extension to modify response headers is not a recommended long-term solution, as it can potentially expose your data to security risks.
Following are the steps to do this:
Install a browser extension that allows you to modify the response headers.
Some popular options include:
- CORS Everywhere (Firefox): Click Here..
- Allow CORS: Access-Control-Allow-Origin (Chrome): Click Here..
Once you have installed the extension, navigate to the web page that is triggering the error.
- Open the browser developer tools by pressing F12 or right-clicking on the page and selecting "Inspect" or "Inspect element".
- Navigate to the "Network" tab in the developer tools and reload the page.
- Find the request that is triggering the error and click on it to view the details.
- In the response headers section, look for the "Access-Control-Allow-Origin" header. If it is not present, this is likely the cause of the error.
- Use the browser extension to modify the response headers by adding an "Access-Control-Allow-Origin" header. Set the header value to "*" to allow any domain to access the resource, or set it to the domain of your web page to restrict access to specific domains.
Reload the page and try the request again. The "No 'Access-Control-Allow-Origin' header is present on the requested resource" error should now be resolved.
It's important to note that using a browser extension to modify response headers is not a recommended long-term solution, as it can potentially expose your data to security risks. It's best to fix the issue on the server-side by properly setting the "Access-Control-Allow-Origin" header in the server response.
What is Cross-Origin Resource Sharing (CORS)?
CORS stands for Cross-Origin Resource Sharing. It is a security feature implemented by web browsers that allows web pages to make XMLHttpRequest (XHR) requests to resources (such as data, scripts, and images) hosted on a different domain or origin, in a secure and controlled manner.
Under the same-origin policy, web pages are only allowed to access resources that originate from the same domain as the web page itself. However, in modern web development, it is often necessary for web pages to access resources hosted on a different domain, such as APIs or web services.
CORS allows web servers to explicitly grant access to their resources from web pages hosted on a different domain. When a web page makes a cross-origin XHR request, the browser sends an HTTP request to the server with an additional "Origin" header, indicating the domain of the web page making the request. The server can then respond with an "Access-Control-Allow-Origin" header, indicating which domains are allowed to access the requested resource.
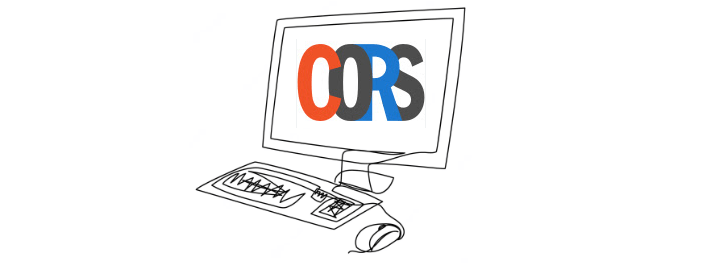
For example, if a web page hosted on "https://example.com" makes a cross-origin XHR request to an API hosted on "https://api.example.org", the browser sends an HTTP request to the API with the "Origin: https://example.com" header. The API can then respond with the "Access-Control-Allow-Origin: https://example.com" header, indicating that the requested resource is allowed to be accessed by web pages hosted on "https://example.com".
CORS provides a secure way for web pages to access resources hosted on a different domain, while still enforcing the same-origin policy to prevent unauthorized access to sensitive data.
What is Same-Origin Policy (SOP)?
The same-origin policy is a security mechanism implemented by web browsers to prevent web pages from making unauthorized requests to resources from a different origin. An origin is defined by the combination of the protocol, domain name, and port number of a web page.
Under the same-origin policy, a web page can only access resources from the same origin as the web page itself. This means that JavaScript running on a page from one origin cannot access resources from a different origin, such as cookies, local storage, or other data. This is done to prevent malicious actors from using a website to access sensitive data or perform actions on behalf of the user without their consent.
However, there are some ways to relax the same-origin policy. For example, cross-origin resource sharing (CORS) can be used to allow resources from a different origin to be accessed under certain conditions, such as when the server explicitly allows it. Similarly, iframe sandboxing can be used to allow content from different origins to be embedded in a web page, while still limiting the scope of its interactions with the parent page.
Error: Access to fetch at 'https://example.com' from origin 'http://yoursite.com' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resourceConclusion:
The error "No 'Access-Control-Allow-Origin' header is present on the requested resource" occurs when you try to access a resource from a different domain, and the server hosting the resource does not allow cross-origin requests from your domain. You can solve this error by modifying the server response to include the Access-Control-Allow-Origin header, using a proxy server, or using a browser extension.
- Difference between using "let" and "var" in JavaScript
- How to Use Array forEach() in JavaScript
- How to Remove Duplicate Values from a JavaScript Array?
- How To Get a Timestamp in JavaScript
- Function Declarations vs. Function Expressions in JavaScript
- How to append values to an array in JavaScript?
- How To Remove a Property from a JavaScript Object
- Which equals operator (== vs ===) should be used in JavaScript comparisons?
- How to Capitalize the First Letter of Each Word in JavaScript
- Get the size of the screen/browser window | JavaScript/jQuery
- Difference between setInterval and setTimeout in JavaScript
- Can't set headers after they are sent to the client