Remove Duplicate Elements from a JavaScript Array
The JavaScript Array.filter() method creates a new array containing elements from the original array that satisfy a specified condition, determined by the function provided as an argument. This method iterates through each element in the array and invokes the given function on each element. Elements that pass the test (i.e., for which the function returns true) are included in the new array, while elements that fail the test (i.e., for which the function returns false) are excluded.
The resulting new array, therefore, only contains elements that meet the criteria specified by the callback function. This makes Array.filter() a powerful tool for selectively extracting or creating a subset of elements from an array based on specific conditions, promoting a functional and expressive approach to array manipulation in JavaScript.
Above code iterate over the array and, for each element, check if the first position of this element in the array is equal to the current position . Obviously, these two positions are different for duplicate elements.
Using JavaScript Set
The JavaScript Set() method is a valuable tool as it enforces uniqueness among its elements. When you add values to a Set, it automatically ensures that duplicates are not allowed. Any attempt to add a value that already exists in the Set will be ignored, ensuring that only distinct elements are stored in the Set.
This unique feature simplifies the task of managing collections of data by automatically removing duplicates, saving developers the effort of manually handling duplicates in the code. The Set() method is particularly useful when you need to work with a list of items where each item should be unique, such as maintaining a list of unique user IDs, email addresses, or any other data that must be distinct.
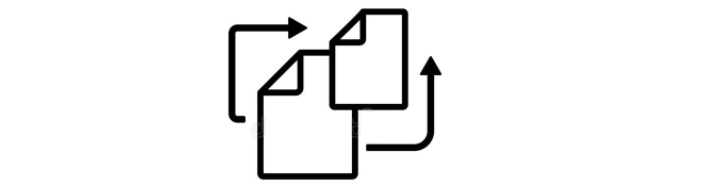
Using JavaScript reduce
The JavaScript reducer, implemented using the reduce() method, systematically iterates through each element of the array, performing a specified operation at each step and gradually accumulating a final result. This iterative process starts by taking the first array element as the initial value and then sequentially combining it with the subsequent array elements, following the defined operation until all items have been processed.
During each iteration, the current array value is added to the result obtained from the previous step, and this cumulative value becomes the new result for the next iteration. This progressive accumulation continues until there are no more elements left to add in the array, ultimately producing a single output that represents the outcome of applying the specified operation to the entire array.
Conclusion
The reduce() method is a powerful and versatile tool in JavaScript for performing various data aggregations, calculations, and transformations on arrays, leading to concise and elegant code solutions for complex tasks involving array manipulation.
- Difference between using "let" and "var" in JavaScript
- How to Use Array forEach() in JavaScript
- How To Get a Timestamp in JavaScript
- Function Declarations vs. Function Expressions in JavaScript
- How to append values to an array in JavaScript?
- How To Remove a Property from a JavaScript Object
- Which equals operator (== vs ===) should be used in JavaScript comparisons?
- How to Capitalize the First Letter of Each Word in JavaScript
- Get the size of the screen/browser window | JavaScript/jQuery
- Difference between setInterval and setTimeout in JavaScript
- Can't set headers after they are sent to the client
- Blocked by CORS policy: No 'Access-Control-Allow-Origin'