Difference Between == and === in JavaScript
In JavaScript, the == and === operators are used for comparison, but they behave differently due to their respective comparison types.
== (Equality Operator)
The == operator checks for equality of values after performing type coercion if the operands have different types. Type coercion is the process of converting one data type to another for comparison. It converts operands to the same type before evaluating the equality.
In this case, the number 1 is converted to a string '1' through type coercion, and the comparison evaluates to true since both operands have the same value.
However, == can lead to unexpected results in certain situations due to its type coercion behavior.
In these cases, the operands have different data types, but the == operator performs type coercion and evaluates the comparison as true.
=== (Strict Equality Operator)
The === operator, also known as the strict equality operator, checks for both equality of values and data types. It does not perform type coercion.
In this case, the === operator evaluates to false because the operands have different data types: number and string.
The strict equality operator is more predictable and generally recommended because it avoids unexpected type coercion. It ensures that both value and data type must match for the comparison to evaluate to true.
In these cases, the === operator evaluates to false since the operands have different data types.
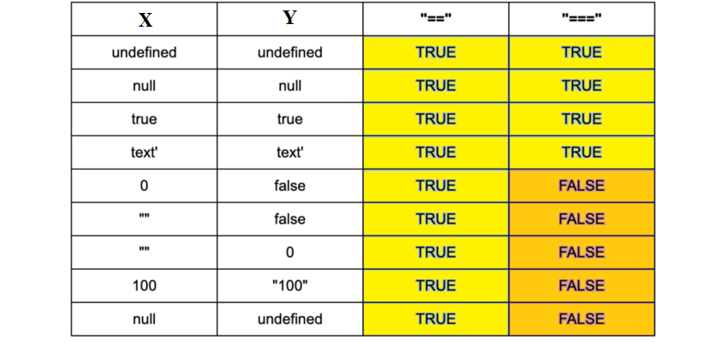
Conclusion
The == performs type coercion before comparison and is less strict, while === does not perform type coercion and is more strict, considering both value and data type for comparison. It is generally recommended to use === (strict equality) as it leads to more predictable and less error-prone code.
- Difference between using "let" and "var" in JavaScript
- How to Use Array forEach() in JavaScript
- How to Remove Duplicate Values from a JavaScript Array?
- How To Get a Timestamp in JavaScript
- Function Declarations vs. Function Expressions in JavaScript
- How to append values to an array in JavaScript?
- How To Remove a Property from a JavaScript Object
- How to Capitalize the First Letter of Each Word in JavaScript
- Get the size of the screen/browser window | JavaScript/jQuery
- Difference between setInterval and setTimeout in JavaScript
- Can't set headers after they are sent to the client
- Blocked by CORS policy: No 'Access-Control-Allow-Origin'