Class Vs Interface in C#
A class in C# serves as a blueprint or prototype that outlines the structure and behavior of objects belonging to the same class category. It provides a specification for constructing individual objects with similar characteristics. Essentially, a class acts as a template that defines the properties, methods, and other members that objects of its type can possess. It encompasses both the state, represented by the properties, and the behavior, represented by the methods, that objects of its type can exhibit. By defining a class, we establish the framework for creating and working with objects that share common attributes and functionalities.
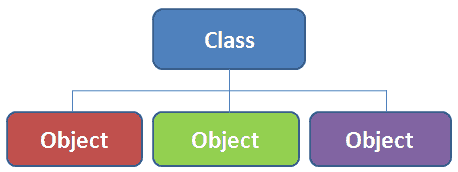
In Object-Oriented Programming (OOP), we can perceive a Toyota car as an instance of the Car class. A class serves as a blueprint or template for creating objects. By utilizing this blueprint, we can construct multiple car objects that adhere to the specifications outlined in the Car class. Each car object represents a unique entity with its own distinct attributes and behaviors. Additionally, each car object is assigned a specific identifier known as its object reference, which enables us to uniquely identify and refer to a particular car object within the program.
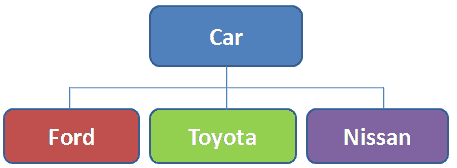

An interface serves as a contract that defines the expected behavior and structure of objects, without specifying the implementation details. It acts as a reference type and solely contains abstract members such as events, methods, and properties, without providing any concrete implementations.
When a class implements an interface, it is obligated by the compiler to provide implementations for all the members defined in the interface. This means that if you choose to implement an interface in your class, you must declare and define all the events, methods, and properties specified by the interface. An interface exclusively consists of abstract methods and constants, which are inherently public, static, and final in nature.
Differences between classes and interfaces
- Implementation: Classes provide both the definition and implementation of members, while interfaces only define the structure of members without any implementation.
- Inheritance: Classes support single inheritance, meaning a class can inherit from only one base class. Interfaces support multiple inheritance, allowing a class to implement multiple interfaces.
- Instantiation: Classes can be instantiated to create objects. Interfaces cannot be instantiated directly but can be implemented by classes, and objects of those classes can be created.
- Access Modifiers: Class members can have different access modifiers like public, private, protected, etc. Interface members are implicitly public and cannot be private.
- Use Cases: Classes are used to create objects with specific properties and behaviors. Interfaces are used to define common behaviors that can be shared across multiple classes, promoting code consistency and reusability.
Conclusion
It is common to use classes and interfaces together to achieve flexible and extensible designs in C# applications. Classes provide concrete implementations, while interfaces define contracts that classes can adhere to, enabling polymorphism and modular design.
- Difference between a Value Type and a Reference Type
- System level Exceptions Vs Application level Exceptions
- Difference between sub-procedure and function
- What does the term immutable mean
- What does the keyword static mean | C#
- this.close() Vs Application.Exit()
- Difference between a.Equals(b) and a == b
- Difference between Hashtable and Dictionary
- Difference between Error and Exception
- C# Dictionary Versus List Lookup Time
- Why does C# doesn't support Multiple inheritance
- How to get the URL of the current page in C# - Asp.Net?