Difference between sub-procedure and function
In programming, sub-procedures and functions are both important constructs used to organize code and perform specific tasks. Although they serve a similar purpose, there are key differences between them in terms of their return values and usage.
Sub-procedure
A sub-procedure, also known as a subroutine or method, is a block of code that performs a specific task or set of tasks. It is typically used for code reusability and to divide complex logic into smaller, more manageable pieces. Sub-procedures do not return a value directly; instead, they are executed for their side effects, such as modifying variables or objects, performing calculations, or executing a series of steps.
Example of a sub-procedure in C#:In the above example, the PrintHello sub-procedure simply prints "Hello!" to the console. It does not return any value, but it performs a specific action.
Function
A function is also a block of code that performs a specific task, but unlike a sub-procedure, it returns a value as a result of its computation. Functions are designed to accept input parameters, process them, and produce a result that can be used in further computations or assigned to a variable.
Example of a function in C#:In the above example, the AddNumbers function takes two integer parameters, a and b, and returns their sum. The result of the addition is returned using the return keyword.
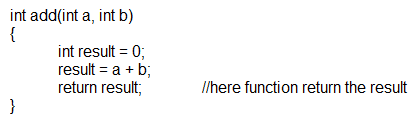
The key differences between sub-procedures and functions can be summarized as follows:
- Return value: Sub-procedures do not return a value, while functions explicitly return a value.
- Usage: Sub-procedures are used for code reusability and performing tasks without needing to return a value, whereas functions are used when a computation or operation needs to produce a specific result.
Here's an example that demonstrates the usage of both a sub-procedure and a function:
In the above example, the Main method calls the PrintHello sub-procedure, which prints "Hello!" to the console. It also calls the AddNumbers function, passing in two numbers, and assigns the returned sum to the sum variable. Finally, the value of sum is printed to the console.
Conclusion
Sub-procedures are used for code reusability and performing tasks without returning a value, while functions are used to perform computations and return a specific result. Both sub-procedures and functions are important building blocks in programming and allow for modular and organized code.
- Difference between a Value Type and a Reference Type
- System level Exceptions Vs Application level Exceptions
- What does the term immutable mean
- What does the keyword static mean | C#
- this.close() Vs Application.Exit()
- Difference between a.Equals(b) and a == b
- Difference between Hashtable and Dictionary
- Difference between Error and Exception
- C# Dictionary Versus List Lookup Time
- Difference between the Class and Interface in C#
- Why does C# doesn't support Multiple inheritance
- How to get the URL of the current page in C# - Asp.Net?