What does the term immutable mean
In the English language, the term "mutable" refers to the ability to change or be modified, while "immutable" denotes the opposite, meaning something that cannot be changed. When we talk about an "immutable object," we are referring to an object whose state or properties remain fixed and unalterable after its creation.
An immutable object is designed in such a way that once it is instantiated or initialized, its internal state cannot be modified. This characteristic of immutability ensures that the object's properties remain constant throughout its lifetime. Immutable objects typically have properties that are set as read-only or constant, preventing any modifications after the initial assignment.
For example, consider an immutable object called Person, which has properties such as Name, Age, and Gender. Once a Person object is created with specific values assigned to its properties, those values cannot be changed. The Name will always remain the same, the Age will not increase or decrease, and the Gender will remain constant. Any attempt to modify these properties will result in a new instance of the Person object being created with the updated values.
Immutability
The concept of immutability is commonly used in programming to ensure data integrity, thread safety, and to simplify code logic. Immutable objects offer benefits such as improved performance, increased reliability, and ease of use in concurrent programming scenarios. They are especially useful in scenarios where you need to share data across multiple threads or where you want to ensure the consistency of an object's state throughout its usage.
By designing objects as immutable, you can rely on their properties remaining constant, making your code more predictable and less prone to bugs caused by unintended modifications. Immutable objects promote a functional programming style and encourage the use of methods that return new instances with modified values rather than directly modifying the existing object.
Is String in .Net immutable ?
Yes, the String class is an example of an immutable type. Immutable objects, including strings, are designed in a way that their state cannot be changed after they are created. Once a string object is instantiated with a specific value, it remains constant throughout its lifetime, and any attempt to modify it actually results in the creation of a new string object with the desired value.
The immutability of strings in .NET brings several benefits. Firstly, it ensures data integrity and consistency. Since strings cannot be modified, you can rely on their values remaining the same, which is crucial in scenarios where data integrity is crucial. Immutable strings also contribute to thread safety, as multiple threads can safely access and use the same string object without the risk of concurrent modifications.
Consider the following example:
In this example, when we concatenate the string "Hello" with ", world!", a new string object is created with the combined value. The original string object "Hello" remains unchanged, and the variable greeting now references the new string object.
The immutability of strings has important implications for memory management and performance. Since strings cannot be modified, the .NET runtime can optimize memory usage by storing a single instance of each unique string value in a string pool. This optimization reduces memory footprint and improves performance by enabling string comparison and sharing of string instances.
Immutable strings also facilitate string manipulation operations, such as substring extraction or concatenation. When performing operations on strings, each operation generates a new string object, leaving the original strings intact. This behavior ensures that the original strings are not accidentally modified during string manipulation.
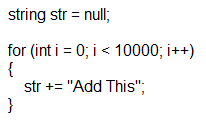
The above code segment results in the creation of 1000 new string variables in the memory.
Multithreaded applications
The immutability of strings offers significant advantages in multithreaded applications by inherently providing automatic thread safety. Since instances of immutable types cannot be modified, they can be safely accessed by multiple threads without the need for additional synchronization mechanisms.
However, the main drawback of immutable types is that they tend to consume more resources compared to other object types. This is because every modification to an immutable object necessitates the creation of a new object instance, resulting in increased memory usage.
StringBuilder class
In situations where frequent modifications to strings are required, such as in the given code segment with 1000 new string variables, it is recommended to utilize the StringBuilder class. Unlike immutable strings, StringBuilder is a mutable type, allowing for modifications to the text without the need to create new instances of the String class. By using StringBuilder, the memory overhead associated with creating multiple string instances can be mitigated, resulting in improved performance and reduced resource consumption.
e.g.
Conclusion
The String class in .NET is immutable, meaning its value cannot be modified after creation. Any modification to a string results in the creation of a new string object. The immutability of strings ensures data integrity, thread safety, memory optimization, and enables efficient string manipulation operations.
- Difference between a Value Type and a Reference Type
- System level Exceptions Vs Application level Exceptions
- Difference between sub-procedure and function
- What does the keyword static mean | C#
- this.close() Vs Application.Exit()
- Difference between a.Equals(b) and a == b
- Difference between Hashtable and Dictionary
- Difference between Error and Exception
- C# Dictionary Versus List Lookup Time
- Difference between the Class and Interface in C#
- Why does C# doesn't support Multiple inheritance
- How to get the URL of the current page in C# - Asp.Net?