Multiple inheritance in C#
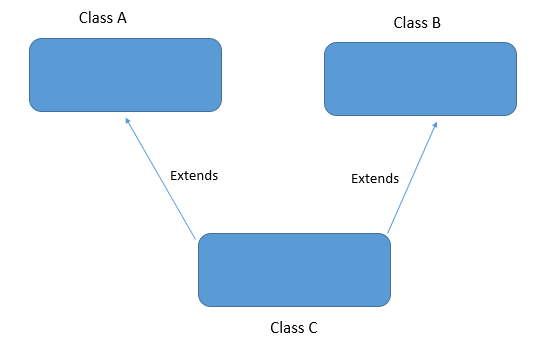

C# does not support multiple inheritance , because they reasoned that adding multiple inheritance added too much complexity to C# while providing too little benefit. In C#, the classes are only allowed to inherit from a single parent class, which is called single inheritance . But you can use interfaces or a combination of one class and interface(s), where interface(s) should be followed by class name in the signature.
Ex:
You can inherit like the following:
In the above code, the class "NewClass" is created from multiple interfaces.
In the above code, the class "NewClass" is created from interface X and class "FirstClass".
WRONG:

The above code is wrong in C# because the new class is created from class "FirstClass" and class "SecondClass". The reason is that C# does not allows multiple inheritance . There are several reasons for this implementation, but it mostly comes down to that multiple inheritance introduces much more complexity into a class hierarchy.
.Net Interface
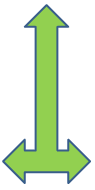
An Interface looks like a class, it contain only the declaration of the members but has no implementation. It provides a contract specifying how to create an Object, without caring about the specifics of how they do the things. An Interface is a reference type and it included only the signatures of methods, properties, events or indexers. In order to implement an interface member, the corresponding member of the implementing class should be public , non-static , and have the same name and signature as the interface member. Click here to know more about.... C# Interfaces .
- Difference between a Value Type and a Reference Type
- System level Exceptions Vs Application level Exceptions
- Difference between sub-procedure and function
- What does the term immutable mean
- What does the keyword static mean | C#
- this.close() Vs Application.Exit()
- Difference between a.Equals(b) and a == b
- Difference between Hashtable and Dictionary
- Difference between Error and Exception
- C# Dictionary Versus List Lookup Time
- Difference between the Class and Interface in C#
- How to get the URL of the current page in C# - Asp.Net?