Value Types and Reference Types
Types are categorized as either Value Types or Reference Types, each with distinct memory allocation behaviors. Value Types encapsulate and store data directly within their own memory allocation, while Reference Types contain a pointer that refers to another memory location where the actual data is stored. It is important to note that Reference Type variables are stored in the heap, a region of memory used for dynamic memory allocation, while Value Type variables are stored in the stack, a region of memory used for local variable storage and function call operations.
By classifying types into Value Types and Reference Types, the .NET Framework effectively manages memory allocation and storage mechanisms. Value Types provide efficient memory usage by directly storing data, making them suitable for smaller and frequently used variables. On the other hand, Reference Types offer flexibility and support complex data structures by storing references to dynamically allocated memory locations, enabling objects to be shared and accessed across different parts of a program.
Understanding the distinction between Value Types and Reference Types in the .NET Framework is crucial for efficient memory management and optimizing performance. By utilizing the appropriate type based on the specific requirements of a variable or object, developers can effectively control memory allocation and enhance the overall execution of their applications.
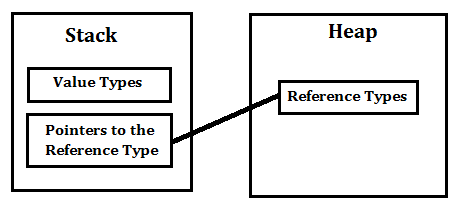
Value Type:
A Value Type represents a data type that stores its contents in memory allocated on the stack. When a Value Type is created, a dedicated space in memory is allocated to hold the value, and the variable directly contains that specific value. If you assign a Value Type to another variable, the value is copied directly, resulting in both variables functioning independently, each with its own separate copy. It's worth noting that predefined data types, structures, and enumerations are also considered Value Types and follow the same underlying behavior.
Value Types are typically created at compile time and stored in stack memory. This allocation on the stack provides efficient memory management and quick access to the values. However, it's important to highlight that the stack memory is not accessible by the garbage collector. Unlike heap memory, which is managed by the garbage collector to reclaim unused objects, the stack memory is not subject to garbage collection. This means that Value Types have a limited lifetime and are automatically deallocated once they go out of scope.
exampleHere the value 10 is stored in an area of memory called the stack.
Reference Type:
Reference Types are employed by a reference that holds the address (reference) of an object rather than the object itself. Unlike Value Types, which store the actual data directly, Reference Types represent the memory address where the data is stored. Consequently, when a reference variable is assigned to another, the data is not copied. Instead, a new reference is created, pointing to the same memory location on the heap as the original value.
Reference Type variables, such as Classes, Objects, Arrays, Indexers, and Interfaces, are stored in a distinct area of memory called the heap. This memory region allows for flexible allocation and deallocation of objects during runtime. When a reference type variable is no longer in use, it can be marked for garbage collection. The garbage collector periodically identifies and releases memory occupied by unreferenced objects, ensuring efficient memory management within the heap.
exampleIn the above code the space required for the 20 integers that make up the array is allocated on the heap.
key differences between Value Types and Reference Types
Here are the key differences between Value Types and Reference Types:
Value Types:
- Value Types store their data directly in memory allocated on the stack.
- Each instance of a Value Type holds its own copy of the data.
- Assigning a Value Type to another variable creates an independent copy of the value.
- Value Types are typically predefined data types, structures, and enumerations.
- Value Types are created at compile time and stored in stack memory.
- Value Types have a limited lifetime and are deallocated automatically when they go out of scope.
- Value Types are passed by value when used as method arguments or assigned to new variables.
Reference Types:
- Reference Types store a reference (memory address) to the object rather than the data itself.
- Multiple variables can refer to the same object in memory.
- Assigning a Reference Type to another variable creates a new reference, not a new copy of the data.
- Reference Types include classes, objects, arrays, indexers, and interfaces.
- Reference Types are created at runtime and stored in the heap memory.
- Reference Types are managed by the garbage collector and can be marked for garbage collection when no longer in use.
- Reference Types are passed by reference when used as method arguments or assigned to new variables.
Conclusion
Understanding the distinction between Reference Types and Value Types is crucial in developing robust and memory-efficient applications. By using the appropriate type based on the nature of the data and its usage requirements, developers can optimize memory usage and ensure proper object management within the .NET Framework.
- System level Exceptions Vs Application level Exceptions
- Difference between sub-procedure and function
- What does the term immutable mean
- What does the keyword static mean | C#
- this.close() Vs Application.Exit()
- Difference between a.Equals(b) and a == b
- Difference between Hashtable and Dictionary
- Difference between Error and Exception
- C# Dictionary Versus List Lookup Time
- Difference between the Class and Interface in C#
- Why does C# doesn't support Multiple inheritance
- How to get the URL of the current page in C# - Asp.Net?