jQuery Selectors
The jQuery selector serves as a potent tool facilitating the discovery of DOM elements within your webpage. Typically, your initiation into this process involves invoking the selector function $() within the jQuery framework. These selectors assume the key role of "locators," enabling the identification and retrieval of HTML elements predicated on a spectrum of attributes including names, IDs, classes, types, and attribute values, among other discerning criteria. Akin to the CSS conventions, these selectors expedite the task of web authors, affording them the ability to promptly and effortlessly discern specific clusters of page components for manipulation through the array of methods offered by the jQuery library.
jQuery selectors start with the dollar sign and parentheses - $() . A jQuery statement typically follows the syntax pattern:
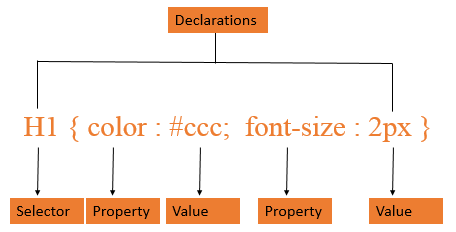
To find elements with a specific class, write a period character , followed by the name of the class:
jQuery Basic Selectors
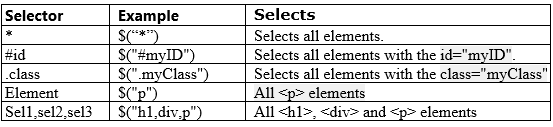
All Selector ("*")
To select all elements of the page, we can use all selectors, for that we need to use * (asterisk symbol).
exampleElement Selector
To select all elements of specific type , we can use element selector. We need to use the html tag name.

This is a Paragraph
The above code shows how to change the content of a paragraph with new content.
Full SourceSelecting Elements by ID
Each element possesses a distinctive and singular ID within the confines of a web page. The hash (#) operator assumes the key role of facilitating the selection of elements predicated on their unique ID attributes. Through the utilization of the id selector pattern, you gain the ability to pinpoint a specific element with precision.
The above code shows how to append string to the existing in the paragraph element using jQuery ID selector .

I am Here...
Selecting Elements by Class
The jQuery class selector is instrumental in identifying elements that bear a designated class. When seeking out elements associated with a particular class, employ the period character followed by the class name to pinpoint these specific elements.
The above code shows how to append string to the existing in the paragraph element using jQuery Class selector.

I am Here...
Multiple Selectors
It is possible to designate an array of selectors for amalgamation into a unified outcome. However, it's crucial to note that the sequence of DOM elements within the jQuery object may not necessarily mirror the original order.

Paragraph with Class selector
Paragraph with ID selector
Select Elements by Attribute
jQuery further extends its capabilities by enabling the retrieval of elements predicated on the attributes assigned to them. This is accomplished through the utilization of the attr(properties) method, which facilitates the assignment of a key/value object as properties to all elements that correspond to the specified criteria.
SyntaxjQuery empowers us with the capability to adeptly manipulate the properties of HTML elements. Following the acquisition of access to these properties, we retain the flexibility to effect alterations to the attributes at a later juncture.
The above code use jQuery to change the image source, the alt attribute, the title attribute and add additional border attributes to image.


Conclusion
jQuery selectors provide a powerful mechanism for identifying and selecting HTML elements based on various criteria such as classes, IDs, and attributes. These selectors enable efficient manipulation and modification of element properties, granting developers the flexibility to work with specific elements within a webpage.