Traverses the DOM
The DOM serves as the bedrock upon which JavaScript operates within the browser and web documents. This interface forms the conduit through which interaction with webpage content transpires, underscoring the necessity of adept navigation within this model. The practice of traversing the DOM entails moving through it to locate HTML elements (DOM Nodes) based on their positional relationships with other elements. Commencing from a selected element, this approach involves progressive movement until the intended elements are reached, facilitating efficient and targeted access to desired parts of the document.
Document Object
At the core of the DOM structure lies the document object, serving as the origin point for every node within the DOM. The DOM conceptualizes the HTML page in a tree format, akin to a "family tree" illustrating lineage. Proficiency in maneuvering both upwards and downwards within this hierarchical tree, traversing branches, is essential in comprehending the interplay between JavaScript and HTML. This tree structure is anchored by the document object, acting as the root element, characterized by its relationships as a parent, child, or sibling within the tree's arrangement. Each constituent element of this structure is denoted as a Node, collectively forming the building blocks of the DOM.
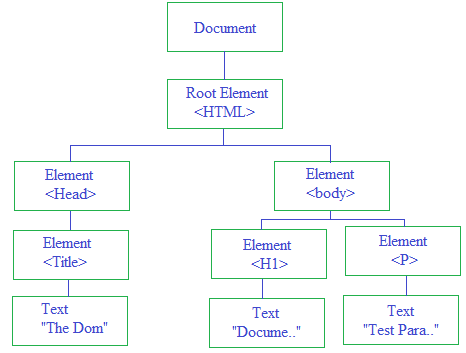
DOM Nodes
Each node present within the DOM tree corresponds to an object that represents a distinct element on the web page. Nodes possess an intrinsic awareness of their connections with neighboring nodes and encapsulate an array of self-descriptive information. The parent node of any given node occupies the tier immediately above it in the DOM hierarchy, while the children nodes are positioned one level below. Nodes that share the same hierarchical level are termed siblings. Every property, apart from childNodes, points to another node object. The childNodes property, conversely, references an array encompassing nodes. Several established methods exist for selecting one or multiple nodes within an HTML document, facilitating tailored node manipulation. The three most popular are:
- getElementById
- getElementsByClassName
- getElementsByTagName

Days:
- Sunday
- Monday
- Tuesday
- Wednesday
The childNodes property furnishes a collection encompassing the child nodes of a specific node, complemented by the length property which indicates the count of these child nodes. Employing a loop, one can iteratively traverse through all the child nodes, thereby accessing and retrieving pertinent information from each child node.

Days:
- Sunday
- Monday
- Tuesday
- Wednesday
Conclusion
Traversing the DOM involves moving through the hierarchical structure of nodes representing elements on a web page. It includes navigating upwards to parent nodes, downwards to child nodes, and horizontally to sibling nodes, while utilizing methods and properties to access and manipulate the relationships and content of these nodes.