Python Tutorial
Python, an influential high-level, object-oriented programming language, was developed by Guido van Rossum and initially introduced in 1991. Its name pays homage to the renowned television series "Monty Python's Flying Circus," and it is commonplace to encounter Monty Python references in example code. Today, Python stands as one of the most widely adopted languages, firmly establishing its position among the most popular programming languages since 2003, as consistently measured by the TIOBE Programming Community Index.
Clean Syntax and Readability
Python boasts a remarkably clean syntax that prioritizes readability, making it easy to understand and write code. Its emphasis on using standard English keywords, combined with well-defined indentation rules, contributes to the clarity and elegance of Python code. This characteristic makes Python an ideal choice for beginners as well as seasoned developers.
Accessible to Programmers of All Backgrounds
Having a foundational understanding of any programming language provides a valuable advantage when learning Python. The language's design philosophy and straightforward syntax facilitate a smooth transition for developers experienced in other programming languages. With its intuitive and concise structure, experienced programmers can quickly grasp Python's concepts and conventions, accelerating their proficiency in the language.
Widespread Popularity and Community Support
Python's popularity has surged over the years, attracting a thriving community of developers and enthusiasts. This extensive support network ensures a wealth of resources , libraries, frameworks, and online forums, making it easier to find solutions, share knowledge, and collaborate on projects. The broad adoption of Python across various industries and domains further solidifies its relevance and viability as a powerful programming language.
A Versatile Cross-Platform Programming Language
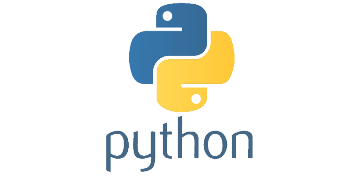
Python, as a cross-platform programming language, exhibits the ability to run seamlessly on multiple platforms, encompassing Windows, Mac OS X, Linux, Unix, and even being ported to the Java and .NET virtual machines. Python interpreters have been developed for a wide range of operating systems, enabling Python code to be executed on diverse systems. Notably, most Python implementations, including CPython, incorporate a read-eval-print loop (REPL) functionality, functioning as a command-line interpreter where users input statements sequentially and receive immediate results. Furthermore, Python's design embraces elements of functional programming, reminiscent of the Lisp tradition, offering support for this paradigm.
Discover the Power and Enjoyment of Python Programming
Python, undoubtedly, stands as an exhilarating and formidable programming language. It strikes a perfect balance between performance and a rich array of features, culminating in a programming experience that is both enjoyable and effortless. As you embark on the forthcoming lessons, you will have the opportunity to explore deeper into the intricacies of Python, unraveling its capabilities and unlocking the potential for creating impressive programs. Prepare to embark on an exciting journey and expand your knowledge of the remarkable world of Python.
Related Topics
- Introduction to Python Programming
- Python Fundamentals
- Python Control Flow Statements
- Python Data Structures
- File and Directory Operations Using Python
- Python Essentials
- Python Exercises
- Python Networking Programming
- Python Programming
- Python Errors and Exceptions
- Top Python Interview Questions (2024)
- Python - Sitemap