Python Exercises
Python is widely acknowledged for its approachable nature, rendering it a particularly accessible programming language, particularly for individuals at the novice level. The language's innate simplicity and lucid syntax contribute to its status as an optimal choice for aspiring developers, as it alleviates the initial hurdles associated with learning to code.
Python resources
Python enjoys the distinct advantage of boasting a vibrant and dynamic community, promoting an abundance of tutorials, forums, and diverse resources that cater specifically to novice learners. These invaluable assets streamline the learning process, ensuring swift and seamless acquisition of coding skills. Moreover, Python's versatility extends far beyond basic programming, as it caters to an extensive range of applications, including web scraping, data visualization, and the rapidly expanding domain of machine learning, thereby solidifying its position as a language that empowers users to explore and accomplish a plethora of advanced tasks.
Python Examples
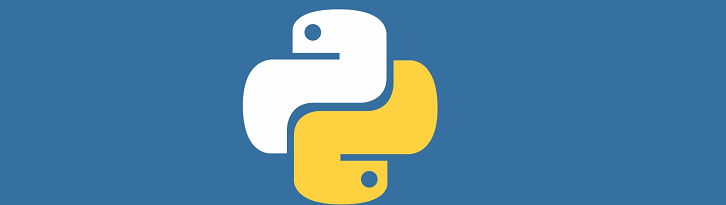
Here are a few examples of Python code that demonstrate some of its basic features:
Python Hello World
Python Variables
Python Conditionals
Python for Loop
Python while Loop
Python Functions
Python Classes
These are just a few examples of the many things that can be done with Python. It is a very versatile programming language and can be used for a wide variety of tasks.
Mastering Python, like any programming language, requires dedication and persistence. Proficiency cannot be achieved overnight, but rather through consistent practice and perseverance. Nonetheless, investing time and effort in learning Python can prove immensely rewarding, as it equips individuals with a valuable and versatile skill set.
Conclusion
To facilitate the learning journey, a prudent step is to set up a Python development environment, such as Anaconda, which streamlines the management of various Python versions and packages. This ensures a seamless and efficient coding experience, allowing learners to focus on honing their programming expertise without being bogged down by setup complexities.
- Print the following pattern using python
- Python Program to Check Leap Year
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- How to Use Modulo Operator in Python
- Enumerate() in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to Convert List to String in Python
- How to convert int to string in Python
- Random Float numbers in Python