Singleton Design Pattern in | C# Implementation
The Singleton Design Pattern is a creational pattern that ensures that a class has only one instance, and provides a global point of access to that instance. In other words, it is a pattern that restricts the instantiation of a class to a single object, and provides a way to access that object from anywhere in the application.
The Singleton pattern is typically used in situations where there is a need to ensure that there is only one instance of a class, and that instance needs to be shared across the application. Examples of such situations include configuration settings, logging, caching, and database connections.
Singleton Pattern
The basic idea behind the Singleton pattern is to define a private constructor for the class, which prevents the creation of new instances of the class from outside the class. Instead, the class provides a public static method or property that returns the single instance of the class. This method or property creates the instance if it doesn't already exist, and returns the same instance on subsequent calls.
One of the main benefits of using the Singleton pattern is that it provides a way to centralize the management of a shared resource or service, which can help to improve the efficiency, scalability, and maintainability of the application. It also ensures that the state of the singleton instance is consistent across the application, since all access to the instance goes through the same public method or property.
Singleton Class Structure
- Private static field to hold the single instance of the class.
- Private constructor to prevent the creation of instances from outside the class.
- Public static property to provide global access to the single instance.
- Public methods and properties to provide the functionality of the class.
A Singleton class typically look like the following:
In above structure, the Singleton class has a private constructor to prevent the creation of instances from outside the class, a private static field to hold the single instance of the class, and a public static property to provide global access to the single instance.
The Instance property creates the instance of the class when it is first accessed, and returns the same instance on subsequent accesses. The class can also have public methods and properties to provide the functionality of the class, as well as other members as needed.
It's important to note that this is just a basic structure for a Singleton class, and it can be modified and customized to suit the specific needs of the application. Additionally, there are different ways to implement the Singleton pattern, and the structure may vary depending on the implementation approach.
How to Implement Singleton Pattern in C# code
In C#, the Singleton Pattern can be implemented in several ways. Following is the full C# code for the program that implements the Singleton pattern using a static constructor:
Note that this implementation uses a private constructor and a static Instance property to ensure that only one instance of the Singleton class is created and accessed throughout the application. The SayHello() method is just a simple example of how the class can be used.
The Singleton pattern is a design pattern that ensures a class has only one instance and provides a global point of access to that instance.
Ways to implement the Singleton pattern in C#
In C#, there are several ways to implement the Singleton pattern:
- No Thread Safe Singleton:This implementation creates an instance of the class on the first call to the GetInstance() method. It is not thread-safe because multiple threads can create multiple instances of the Singleton, resulting in inconsistent behavior.
- Thread-Safety Singleton:This implementation uses the lock statement to ensure that only one thread at a time can create an instance of the Singleton. While it is thread-safe, it can be slow if many threads try to access the Singleton concurrently.
- Thread-Safety Singleton using Double-Check Locking:This implementation checks if an instance of the Singleton has been created before acquiring the lock and creating the instance. This can improve performance by avoiding the lock when an instance already exists.
- Thread-safe without a lock:This implementation uses the volatile keyword to ensure that multiple threads can safely access the Singleton without a lock. It is thread-safe and performs better than the previous implementation, but it is not completely foolproof.
- Using .NET 4's Lazy
type: This implementation uses the Lazyclass from the .NET framework to ensure that the Singleton is created only when it is first accessed. It is thread-safe and performs well, but it requires .NET 4 or later.
Each implementation has its advantages and disadvantages, and the choice depends on the specific requirements of the application.
Thread-safe Singleton class using the double-checked locking
Following is an example of a thread-safe Singleton class implemented in C# using the double-checked locking technique:
In the above implementation, use the volatile keyword to ensure that the instance field is always read from and written to the main memory, and not from a cache. Also use a lock statement to ensure that only one thread can access the critical section of code that creates the Singleton instance.
Double-checked locking technique
The double-checked locking technique is used to avoid the overhead of acquiring a lock on every access to the Instance property. The first check outside the lock statement verifies if the instance is null, and if it is, then the thread enters the lock statement for further verification. Once the thread acquires the lock, it checks the instance field again to make sure that no other thread has already created an instance, and then creates a new instance if necessary.
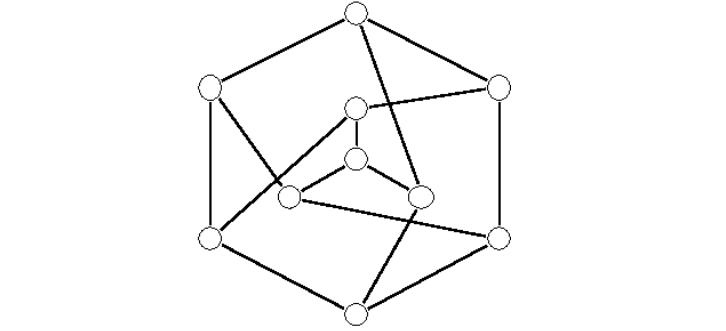
To use the Singleton class in your application, you can simply call the Instance property to obtain the single instance of the class, and then use its public methods and properties as needed. Following is the code of how to use it:
This will create the singleton instance if it doesn't already exist, and then call the SayHello method on the singleton instance, which will output "Hello from Singleton!" to the console.
Advantages of Singleton design pattern
The Singleton design pattern offers several advantages:
- Ensures a single instance: The primary advantage of the Singleton pattern is that it ensures that only one instance of a class exists in the system. This can be important in situations where having multiple instances could cause conflicts or waste resources.
- Global access: The Singleton instance can be globally accessed from anywhere in the codebase. This means that it's easy to call its methods and access its properties without having to create an instance of the class each time.
- Lazy instantiation: With a Singleton, the instance is only created when it's needed. This can be important in situations where the initialization of the instance is expensive or time-consuming.
- Controlled access: The Singleton pattern allows you to control access to the instance by providing a single point of access to it. This can be useful for managing resources or enforcing business rules.
- Compatibility with other patterns: The Singleton pattern is often used in conjunction with other patterns such as the Factory Method or the Command pattern. This compatibility can make it easier to implement complex systems.
Disadvantages of Singleton Design Pattern
Although the Singleton pattern provides several benefits, there are also some potential disadvantages to consider when using it:
- Tight coupling: The Singleton pattern can create tight coupling between classes, which can make the code less modular and harder to maintain. Because the Singleton instance is globally accessible, it can be difficult to change or replace without affecting other parts of the system.
- Global state: Since the Singleton instance is globally accessible, it can potentially lead to global state in the system, which can make it harder to reason about the behavior of the code. Global state can make it difficult to test, debug, or modify code, especially in large systems.
- Thread safety: Without proper implementation, the Singleton pattern can be vulnerable to race conditions and other concurrency issues. It's important to ensure that the Singleton instance is thread-safe to avoid unexpected behavior or crashes.
- Difficulty with testing: Due to the tight coupling and global state, testing code that relies on a Singleton can be more challenging. It may be difficult to isolate the code being tested or to mock the Singleton instance.
- Hidden dependencies: Because the Singleton instance is not explicitly passed around as a dependency, it can create hidden dependencies in the code. This can make it harder to understand the relationships between classes and modules.
Conclusion:
The Singleton pattern can be a useful tool, but it should be used with care and consideration. It's important to weigh the benefits against the potential drawbacks and to ensure that the pattern is implemented properly.