Foreach Loop in C#
The foreach loop in C# is used to iterate over a collection of objects, such as an array, a list, or any other collection that implements the IEnumerable interface. The basic syntax of the foreach loop is:
Syntax:The foreach loop is a convenient way to iterate over a collection of objects without having to manually manage an index or iterator variable. It also helps to make your code more readable and concise.
In C#, you can use the foreach loop to iterate over elements in an array, a collection, or any other enumerable object.
C# Array using foreach loop
In the above example, use the foreach loop to iterate over each element in the array and print it to the console.
C# List using foreach loop
In the above example, use the foreach loop to iterate over each element in the list and print it to the console.
It's important to note that the foreach loop is read-only, which means you cannot modify the elements in the collection while iterating over them. If you need to modify the collection, you should use a regular for loop instead.
C# Foreach loop in Dictionary
In C#, you can use a foreach loop to iterate over the key-value pairs in a dictionary. The foreach loop will iterate over the keys of the dictionary, and you can use the keys to access the corresponding values.
In the above example, there is a dictionary called colors that maps strings (colors) to integers (values). Inside the foreach loop, use the Keys property of the dictionary to iterate over the keys of the dictionary, which are the colors. Inside the loop, use the key to access the corresponding value using the square bracket notation, and then print the color and value to the console.
Notice that you're able to iterate over the key-value pairs in the dictionary using a foreach loop by iterating over the keys and then accessing the corresponding values using the keys. If you need to iterate over both the keys and the values, you can use a foreach loop with the KeyValuePair type, like this:
In the above example, use a foreach loop with the KeyValuePair
Foreach Loop skip to next item
In C#, you can use the continue statement to skip the current iteration of a foreach loop and move on to the next iteration. When the continue statement is encountered inside a foreach loop, the loop immediately jumps to the next iteration and skips over any remaining code in the current iteration.
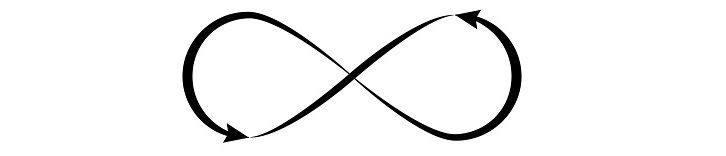
Following is an example that uses the continue statement to skip over elements that meet a certain condition:
In the above example, there is an array of strings called colors. Use a foreach loop to iterate over each element in the array. Inside the loop, also use an if statement to check if the length of the current element is >5. If it is, use the continue statement to skip over the rest of the code in the current iteration and move on to the next iteration. If the length is less than 5, print the current element to the console. Notice that the loop skipped over the elements "no-color" because it has a length of >5.
Foreach Loop - break
In C#, you can use the break statement to exit a foreach loop prematurely. When the break statement is encountered inside a foreach loop, the loop is immediately terminated and control is transferred to the statement following the loop.
In the above example, there is an array of integers called numbers. Use a foreach loop to iterate over each element in the array. Inside the loop, use an if statement to check if the current element is equal to 300. If it is, use the break statement to exit the loop prematurely. If it's not, print the current element to the console. Notice that the loop terminated early when it encountered the element with the value 3.
Get the index of the current iteration of a foreach
In C#, you can use the foreach loop to iterate over the elements of an array or collection, but it doesn't provide an explicit way to get the index of the current iteration. However, you can use a separate counter variable to keep track of the index yourself.
In the above example, there is an array of strings called colors. Use a separate integer variable called index to keep track of the index of the current iteration. Inside the loop, print the index and the current element to the console, and then increment the index variable.
Alternatively, you can use the Array.IndexOf method to get the index of the current element in the array.
In the above example, use the Array.IndexOf method to get the index of the current element in the colors array. Inside the loop, print the index and the current element to the console.