What are the Default Access Modifiers in C#?
In C#, default access modifiers are used when an explicit access modifier is not specified for a type or type member. The default access modifiers vary depending on the context in which they are used. Let's explore the default access modifiers for different elements in C#:
Class and Struct Members
For class and struct members (fields, methods, properties, and events), the default access modifier is private. This means that if no access modifier is explicitly specified, the member will be accessible only within the same class or struct.
In the above example, the myField field and MyMethod() method have the default access modifier of private. They can only be accessed within the MyClass class.
Class and Struct
For classes and structs themselves, the default access modifier is internal. This means that if no access modifier is specified, the class or struct will be accessible only within the same assembly (or project in Visual Studio).
In the above example, the MyClass class has the default access modifier of internal, making it accessible only within the same assembly.
Interfaces and Enumerations
Interfaces and enumerations have different default access modifiers:
Interfaces: The default access modifier for interfaces is public. If no access modifier is specified, the interface is accessible from any code that can access the declaring namespace or assembly.
In the above example, the IMyInterface interface has the default access modifier of public.
Enumerations: The default access modifier for enumerations (enums) is also public. If no access modifier is specified, the enumeration is accessible from any code that can access the declaring namespace or assembly.
In the above example, the MyEnum enumeration has the default access modifier of public.
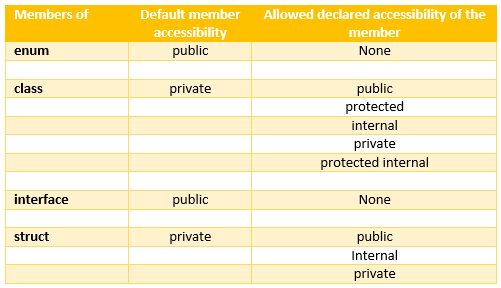
Conclusion
It's important to note that the default access modifiers can be overridden by explicitly specifying a different access modifier. Using explicit access modifiers is recommended for clarity and to ensure proper encapsulation and access control in your code.