What is operator overloading in c#?
Operator overloading in C# allows developers to define custom implementations for operators when one or both operands are of user-defined class or struct types. Overloaded operators are special functions denoted by the "operator" keyword followed by the symbol of the operator being defined. Similar to regular functions, overloaded operators have return types and parameter lists. This feature sets C# apart from other languages like Java, making it particularly appealing to programmers working on large libraries or frameworks.
Overloading operators
By overloading operators, you can redefine or modify the behavior of most built-in operators in C#, enabling their usage with user-defined types. Let's consider an example to illustrate this concept. Suppose we have a class called "Box" with properties for height and width. We create three instances of the Box class: box_1, box_2, and sizeOfBoxes.
Now, let's say we want to add the sizes of two boxes and assign the result to the third box. If we simply try to use the "+" operator to add the two boxes and assign the result to the third box, it would result in an error since the "+" operator is not defined for the Box class by default.
To address this, we can overload the "+" operator for the Box class, specifying how the addition operation should be performed for two Box objects. By providing a custom implementation for the "+" operator, we can define the logic for adding the sizes of two boxes and correctly assign the result to the third box.
Because you can not add two object with plus operator. You have to overload the operator to define the task of "+" operator within the class when two operand is instance of rectangle class.
ExampleThe above function implements the addition operator (+) for a user-defined class Box. It adds the attributes of two Box objects and returns the resultant Box object. Now you can make a call to overloaded operator method from where it is accessible. Not all operators can be overloaded, however, and others have restrictions, as listed in this table:
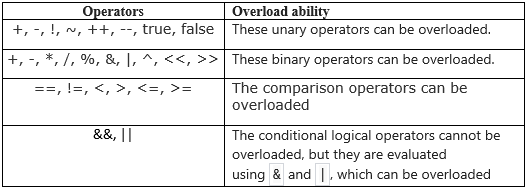
While operator overloading can make code concise and expressive, it can also introduce ambiguity or confusion for users who are not familiar with the specific operator implementations. In such cases, providing equivalent methods alongside the overloaded operators can greatly enhance code clarity and usability.
By offering alternative methods that accomplish the same functionality as the overloaded operators, users who may find the operators unintuitive or unclear can resort to using the methods instead. These methods can have descriptive names that clearly indicate their purpose, making them more self-explanatory and easier to understand.
By providing both overloaded operators and equivalent methods, you offer users multiple options for achieving the desired results, catering to different preferences and levels of familiarity with the operators. This approach enhances the usability of your library or codebase and allows users to choose the style that best suits their needs and comprehension.
It is important to consider the needs and expectations of your users and strike a balance between the convenience of operator overloading and the clarity provided by alternative methods. By offering both options, you can make your code more accessible and user-friendly, accommodating a wider range of programming styles and preferences.
Conclusion
Using operator overloading, we can extend the functionality of operators to work with user-defined types, providing more flexibility and ease of use. It allows for intuitive and expressive code, making it simpler to work with custom types and perform operations specific to those types.