How to link jQuery from CDN
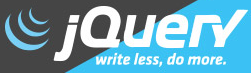
jQuery is an adaptable JavaScript library applicable to various development scenarios. It's characterized by its open source nature, available under the dual licensing of the MIT (Massachusetts Institute of Technology) License and the GNU (GNU's Not UNIX) General Public License. Remarkably, jQuery stands apart from other JavaScript libraries as the most extensively utilized one globally, offering programmers invaluable pre-written JavaScript solutions, thus greatly facilitating their work across a multitude of projects.
jQuery setup
Officially there are two ways of using jQuery:
- CDN Based Version - You can include jQuery library into your HTML code directly from Content Delivery Network (CDN).
- Local Installation - You can download jQuery library on your local machine and include it in your HTML code.
CDN Based Version
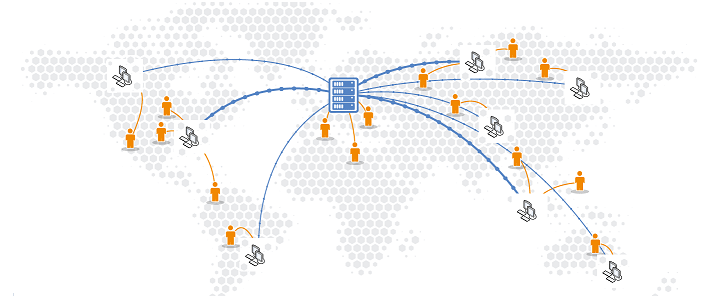
A Content Delivery Network (CDN), also known as a Content Distribution Network, comprises a network of proxy servers distributed globally in various geographical locations. These servers store cached content, allowing end users to efficiently access it. Notably, major technology companies like Google and Microsoft provide hosted copies of jQuery libraries via their CDNs. Employing a CDN can significantly enhance the performance of both your jQuery library and your entire website. To incorporate a hosted jQuery library, you simply need to insert the corresponding HTML code snippet provided below into your HTML page.
Google CDN
Microsoft CDN
We recommend that you load jQuery libraries from the CDN via HTTPS, even if your own web-pages only uses HTTP.
Using a CDN for jQuery yields numerous benefits, foremost among them being the substantial enhancement in loading speed. CDNs are carefully designed for accelerated loading, leading to swift downloads of the jQuery library from the Content Delivery Network. As a result, your web pages experience a noticeable acceleration in loading times, even if it's a matter of mere milliseconds.
Local Installation
There are two versions of jQuery libraries accessible for downloading from websites:
- Production version - this is for your live website because it has been minified and compressed.
- Development version - this is for testing and development (uncompressed and readable code).
The uncompressed version is preferable during programming or debugging; the compressed version saves bandwidth and boost performance in production level. Both versions can be downloaded from jQuery.com web-site.
The jQuery library is a javascript file, and you can reference it directly by adding to your html source file.
It's generally advisable to retain the original file name as it appears when downloaded from CDN sources, as it includes the version of jQuery. Additionally, it's important to acknowledge that many websites possess distinct directories for their JavaScript files. Consequently, if you're incorporating jQuery from a CDN, using the complete file path becomes imperative in order to accurately locate and access the desired resource within the structure of the website's file system.
FullSourceConclusion
To link jQuery from a CDN (Content Delivery Network), it's recommended to maintain the original file name containing the version number, ensuring accurate version usage. Since websites often organize JavaScript files in distinct directories, employing the complete file path is crucial for locating the jQuery resource effectively on the CDN.