Sockets programming in Java
Socket programming is a powerful method for establishing communication channels between multiple applications running on a network. By utilizing sockets, applications can effectively exchange data in a secure and efficient manner, facilitating seamless communication and interaction.
What is socket in computer programming?
A socket serves as an essential endpoint that facilitates bidirectional communication between two programs operating on a network. It acts as an interface through which programs can transmit and receive data across a network connection, adhering to established communication protocols like TCP and UDP. Sockets play a fundamental role in a wide range of networked applications, encompassing web servers, database servers, and game servers, enabling seamless communication between distinct components of these applications.
What is server side socket programming?
Server-side socket programming entails implementing network communication protocols on the server side by utilizing socket APIs. This enables seamless communication between the server and clients. The process involves creating a socket on the server, binding it to a specific port, and actively listening for incoming connections from clients. Once a connection is established, the server can send and receive data to and from the client. Server-side socket programming is frequently employed in the development of network-based applications such as web servers, game servers, and various other networked services. It forms a crucial component of building robust and efficient client-server architectures.
What is client side socket programming?
Client-side socket programming involves implementing network communication protocols on the client side using socket APIs to facilitate communication between the client and server. The process entails creating a socket on the client side and establishing a connection to a server using a designated IP address and port number. Once the connection is established, the client can transmit data to the server and receive data from it. Client-side socket programming is extensively utilized in the development of client applications that connect to various networked services, including web servers, game servers, and other networked applications. It enables seamless interaction between the client and server, facilitating efficient data exchange and communication.
How to Socket programming in java?
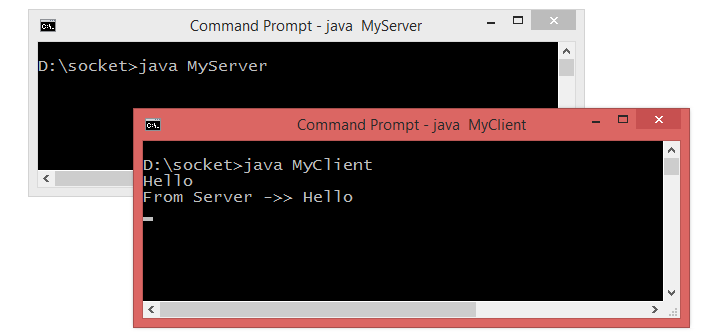
To implement socket programming in Java, you can utilize the java.net package, which offers a collection of classes and methods specifically designed for creating and utilizing sockets. Here are the steps to implement a basic socket program in Java:
How to create a Java Server Socket Program
- Create a server socket on the server side using the ServerSocket class, passing in the desired port number as an argument.
- Listen for incoming connections on the server side using the accept method, which returns a Socket object for the new connection.
- Create an input stream on the server side using the getInputStream method, and read data from the client using the read method.
- Create an output stream on the server side using the getOutputStream method, and write data to the client using the write method.
- Close the sockets on both the client and server sides using the close method.
Java Server side Socket Programming Example
How to create a Java Client Socket Program
- Create a socket on the client side using the Socket class, passing in the server's IP address and port number as arguments.
- Create an output stream on the client side using the getOutputStream method, and write data to the server using the write method.
- Create an input stream on the client side using the getInputStream method, and read data from the server using the read method.
Java Client side Socket Programming Example
In the provided example, the client-side socket programming code demonstrates the following steps:
Create a Socket object: The client creates a Socket object and specifies the server's IP address (localhost) and port number (10007) to establish a connection.
Create output and input streams: The client creates an output stream to send data to the server and a buffered reader to receive data from the server.
Prompt for user input: The client prompts the user to enter data.
Send data to the server: The client writes the user's input to the output stream, which is sent to the server.
Receive and display server response: The client reads the server's response from the input stream and displays it on the screen.
Close the streams and socket: When the user enters null, indicating the end of input, the client closes the input stream, output stream, and socket to release resources.
How to run the server and client socket program in java
To run the server-side and client-side socket programs in Java, you need to have the Java Development Kit (JDK) installed on your machine. You can then compile the code using the javac command and run the compiled code using the java command.
Steps to run the server-side socket program:
- Open a terminal/command prompt window.
- Change the current directory to the location where the server-side code is saved.
- Compile the Java source code using the following command:
- Run the compiled Java code using the following command:
The server will start listening on the specified port for incoming connections.
Steps to run the client-side socket program:
- Open a second terminal or command prompt window.
- Change the current directory to the location where the client-side code is saved.
- Compile the code using the following command:
- Run the compiled Java code using the following command:
The client will connect to the server, and you will be able to send messages to the server and receive responses from it.
Before running the client program, it is important to ensure that the server is running and accessible. Here are a few points to consider:
Start the server program first: Make sure that you have successfully compiled and started the server program. Check the console or terminal window where the server is running for any error messages or indications that the server has started successfully. If there are any errors, resolve them before proceeding.
Verify server accessibility: Ensure that the server is accessible from the machine where you intend to run the client program. If both the server and client are running on the same machine, you can use "localhost" or the loopback IP address (127.0.0.1) as the server address in the client code. However, if the server and client are on different machines, you need to use the IP address or hostname of the server machine.
Check network connectivity: If the server and client are running on different machines, make sure they are connected to the same network. Verify that the machines can communicate with each other by pinging the server machine from the client machine using the command prompt or terminal.
Update client code with server address: If the server and client are on different machines, update the client code to use the IP address or hostname of the server machine. Look for the part in the client code where the server address is specified and modify it accordingly.
Run the client program: Once the server is running and accessible, you can proceed to run the client program. Open a new console or terminal window on the machine where the client is located. Compile the client code if necessary, and then execute the client program using the appropriate java command. Follow the instructions in the client program to interact with the server.
Conclusion
Socket programming in Java allows for reliable and efficient communication between applications over a network, enabling the development of network-based applications such as web servers, game servers, and more.