Java Errors and Exceptions
Both Errors and Exceptions are categorized as subclasses of the java.lang.Throwable class. It is imperative to exercise caution when dealing with Errors, as they generally signify critical issues that should not be caught or handled, except in extremely exceptional scenarios. Conversely, Exceptions serve as the primary focus of exception handling, representing conditions that a reasonable application might actively consider catching and managing in a controlled manner. In summary, Errors demand utmost care and should be avoided in regular exception handling routines, whereas Exceptions play a vital role in the robustness and stability of applications by allowing smooth recovery from anticipated issues.
Errors are exceptional conditions that often signify the irrecoverable state of an application, leading to its termination. They are typically unrecoverable and should prompt the Virtual Machine to exit elegantly. Handling Errors is generally discouraged, except for logging or displaying appropriate messages before the application's closure. JVM usually throws Errors in critical scenarios where the application has no means of recovery, such as OutOfMemoryError, which indicates insufficient memory to execute the program.
There are three kinds of errors:
- Syntax Errors
- Runtime Errors
- Logic Errors
Syntax errors
Syntax errors in Java occur when the compiler identifies issues with the structure of your program, preventing it from being executed. These errors often involve incorrect punctuation, undeclared variables, or other fundamental mistakes in the code. Fortunately, syntax errors are relatively straightforward to detect and rectify, as the compiler provides detailed error messages pinpointing the problematic areas and suggesting potential solutions. This feedback facilitates efficient debugging and ensures that the program adheres to the correct syntax rules, leading to successful compilation and execution.
Runtime errors

Runtime errors, also known as exceptions, occur in a program with correct syntax when the computer encounters an operation or instruction it cannot reliably execute. These errors arise during the program's execution phase and can be caused by various factors such as invalid user inputs, unexpected data, or unavailable system resources. When a runtime error occurs, the program's normal flow is interrupted, and an exception is raised, typically leading to the termination of the program if not properly handled. To ensure robustness and reliability, developers must implement proper exception handling mechanisms to manage these unforeseen scenarios and prevent abrupt program crashes. Common examples are:
- Trying to divide by a variable that contains a value of zero.
- Trying to open a file that doesn't exist.
Runtime errors can be considered intermediate in difficulty when it comes to debugging. While the Java Virtual Machine (JVM) provides valuable information about the location where the error occurred, it requires the developer to trace back from that point to identify the root cause and the origin of the problem. This process involves inspecting the stack trace and understanding the sequence of method calls that led to the runtime error. By carefully analyzing the information provided by the JVM, developers can efficiently pinpoint the source of the issue and implement appropriate fixes to resolve the error, thereby ensuring the smooth and reliable execution of the Java program.
Logic errors
Logic errors, also known as semantic errors, arise when a program's functionality does not align with the intended behavior. Unlike syntax and runtime errors, logic errors do not cause the Java Virtual Machine (JVM) to report specific error messages or provide detailed information about the problem. The JVM executes the program as instructed, regardless of whether the outcomes are correct or not. Identifying and rectifying logic errors require careful inspection of the code, thorough understanding of the program's logic, and a systematic approach to debugging. Developers must employ various techniques, such as code review, using print statements, and employing debugging tools, to detect and resolve logic errors, ensuring the program performs as intended and produces the expected results. Common examples are:
- Multiplying when you should be dividing
- Adding when you should be subtracting
- Opening and using data from the wrong file
- Displaying the wrong message
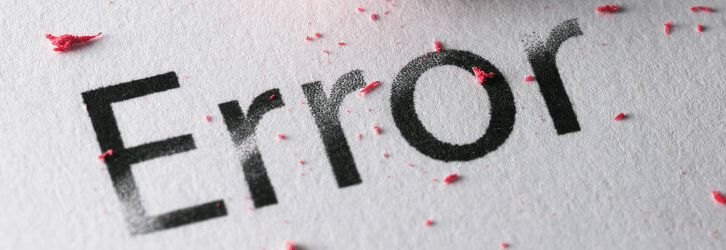
Conclusion
Syntax errors are indeed easy to identify and correct due to the helpful error messages provided by the compiler, indicating the precise location and nature of the issues in the code. Similarly, runtime errors, while causing the program to abort, also display error messages that aid in locating the problem during program execution. However, logic errors present a greater challenge as they do not produce explicit error messages. Instead, they cause the program to behave unexpectedly, making it difficult to pinpoint the root cause of the problem. Detecting logic errors often requires careful debugging, employing various techniques like code inspection, logging, and testing, as well as a solid understanding of the program's intended behavior to isolate and resolve the discrepancies in the code's logic, ensuring the program functions correctly as intended.
- Reached end of file while parsing
- Unreachable statement error in Java
- Int Cannot Be Dereferenced Error - Java
- How to fix java.lang.classcastexception
- How to fix java.util.NoSuchElementException
- How to fix "illegal start of expression" error
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException
- How to handle the ArithmeticException in Java?