Java collections framework
Java Collections are predefined set of classes or data structures which can be used to store multiple items in a single unit. Dynamically allocated data structures in Java (such as Hashtable, HashSet, HashMap,LinkedList, Vector, Stack, ArrayList) are supported in a unified architecture called the Collection Framework, which mandates the common behaviours of all the classes.
For many applications, the developers want to create and manage groups of related objects. There are two options to group objects: by creating arrays of objects, and by creating collections of objects. Collections provide a more flexible way to work with groups of objects. Unlike arrays, these group of objects are powerful and can easily grow or shrink with out having to redefine them. For some collections, you can assign a key to any object that you put into the collection so that you can quickly retrieve the object by using the key. The capacity planning and predefined algorithms for sort, search and manipulation make them easy to use. The array, however, does not support so-called dynamic allocation - it has a fixed length which cannot be changed once allocated. Moreover, array is a simple linear structure. Many applications may require more complex data structure such as linked list, stack, hash table, sets, or trees.
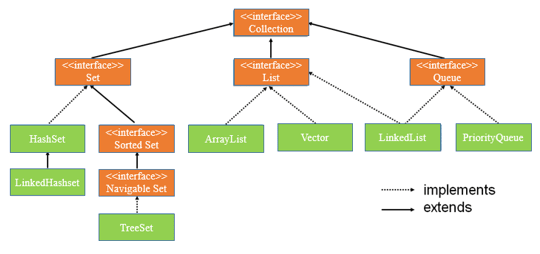
The Java Collection Framework package (java.util) contains:
- Interfaces
- Implementations
- Algorithms
Interfaces
Java Collections Framework interfaces provides the abstract data type to represent collection. The data structures derived from Collection interface are known as collections framework . The java.util.Collection is the root interface of Collections Framework. It is on the top of Collections framework hierarchy. Two main sub interfaces are List and Set . Collection interface methods are overridden by different classes in different ways as per their requirement. Some collection classes do not accept duplicate values like HashSet and TreeSet. The Collection interface comes with many methods so that each class derived can use well. It contains some important methods such as size(), iterator(), add(), remove(), clear() that every Collection class must implement. Map is the only interface that doesn't inherits from Collection interface but it's part of Collections framework. All the collections framework interfaces are present in java.util package.
Implementations
Java Collections framework comes with many implementation classes for the interfaces. We can use them to create different types of collections in java program. Most common implementations are ArrayList, HashMap and HashSet. Some other important collection classes are LinkedList, TreeMap, TreeSet. These are the concrete implementations of the collection interfaces. In essence, they are reusable data structures . These classes solve most of our programming needs but if we need some special collection class, we can extend them to create our custom collection class. Using an existing, common implementation makes your code shorter and quicker to download. Also, using existing Core Java code core ensures that any improvements to the base code will also improve the performance of your code. Java 1.5 came up with thread-safe collection classes that allowed to modify Collections while iterating over it, some of them are CopyOnWriteArrayList, ConcurrentHashMap, CopyOnWriteArraySet. These classes are in java.util.concurrent package. All the collection classes are present in java.util and java.util.concurrent package.
Algorithms
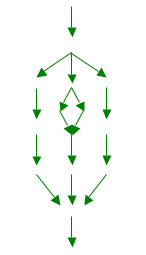
The Collection Framework also provides features to apply different algorithms on all or a few elements of a collection, such as searching through a collection, sorting or shuffling elements of a collection, fetch a read only view of a collection, and so forth. The great majority of the algorithms provided by the Java platform operate on List instances, but a few of them operate on arbitrary Collection instances. The algorithms are said to be polymorphic : that is, the same method can be used on many different implementations of the appropriate collection interface. In essence, algorithms are reusable functionality.
From the following chapters you can learn Java Collections Framework in detail :