What is lazy initialization
Lazy initialization is a design pattern in software development that defers the creation or calculation of an object or value until it is actually needed. Instead of initializing the object or value immediately, lazy initialization delays the initialization process until the first time it is accessed or requested.
Lazy initialization
The main advantage of lazy initialization is improved performance and resource utilization. By postponing the initialization, you avoid unnecessary computation or allocation of resources for objects that might not be used during the execution of the program. This can lead to significant performance gains, especially in scenarios where the initialization is resource-intensive or time-consuming.
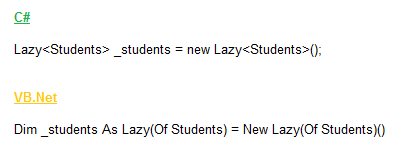
Lazy initialization can be implemented using various techniques, depending on the programming language or framework being used. One common approach is to use a lazy initialization wrapper or a lazy initialization class, which encapsulates the logic for deferring the initialization. This wrapper typically contains a flag or a reference to the initialized object, and upon first access, it checks if the object has been initialized. If not, it performs the initialization and caches the result for subsequent accesses.
Here's an example in C# demonstrating lazy initialization using the Lazy<T>
In the above example, the ExampleClass has a property LazyObject that utilizes lazy initialization. The _lazyObject field is of type Lazy<ExpensiveObject>, and it is initialized with a lambda expression that creates a new ExpensiveObject when accessed for the first time. Subsequent accesses to LazyObject will return the cached instance of ExpensiveObject without re-initialization.
Conclusion
Lazy initialization is particularly useful in scenarios where the creation or initialization of an object is expensive, such as establishing database connections, loading large files, or performing complex calculations. By employing lazy initialization, you can optimize resource usage and improve the overall performance of your application.
- Difference between NameSpace and Assembly
- Execution process for managed code
- What is an Asssembly Qualified Name
- How to Assembly versioning
- Add and remove an assembly from GAC
- What is Native Image Generator (Ngen.exe)?
- How to force Garbage Collection
- Difference between CType and DirectCast
- Obsolete or Deprecated in NET Framework
- Difference between Clone and Copy
- Difference between Finalize() and Dispose()
- Difference between static and constant