Difference between NameSpace and Assembly
Both Namespace and Assembly serve as fundamental concepts that aid in organizing and managing code. However, they have distinct roles and purposes.
Namespace
A Namespace is a logical container that helps in organizing classes, interfaces, structures, enumerations, and other types within a codebase. It provides a hierarchical naming structure to avoid naming conflicts and to facilitate better code organization and modularity. Namespaces enable developers to group related code elements together, making it easier to locate and reference them. They provide a way to organize code based on its functionality or purpose. Multiple namespaces can exist within a single assembly, allowing for better organization and separation of concerns.
Example: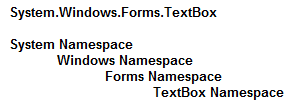
Assembly
An Assembly is a physical unit of deployment that encapsulates compiled code, resources, and metadata required to execute an application or a component. It is a self-contained unit that can be independently deployed, versioned, and distributed. An assembly contains one or more compiled files, such as DLLs (Dynamic Link Libraries) or EXEs (Executable Files), along with additional resources like images, configuration files, and XML documentation. It serves as a building block for applications and provides the necessary information for the runtime to locate and execute the code within it.
Assemblies can be either dynamically linked (DLLs) or executables (EXEs). They can also include one or more namespaces, which help organize the code within the assembly. Each assembly has a unique identity based on its name, version, culture, and public key token, which ensures versioning and compatibility. Assemblies can be private, used by a single application, or shared, where multiple applications can reference and use the same assembly.
Example:Conclusion
While a Namespace provides a logical structure for organizing and grouping code elements within a codebase, an Assembly represents a physical unit of deployment that encapsulates compiled code, resources, and metadata. Namespaces help organize code within an assembly, while assemblies serve as standalone units that can be deployed and executed independently.