FileReader Class in Java
The FileReader class in Java is used to read character data from a file. It provides a convenient way to read characters from a file in a simple and efficient manner. Let's explore its usage and features with examples:
Basic Usage
To use the FileReader class, you need to create an instance of it and pass the file path as a parameter to the constructor. You can then read characters from the file using various read methods provided by the FileReader class.
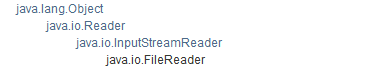
Reading Characters in Bulk
The FileReader class provides a more efficient way to read characters in bulk by using a character array buffer. This can be achieved using the read(char[] cbuf) method.
Handling FileReader with Try-With-Resources
Starting from Java 7, it is recommended to use try-with-resources to manage resources like FileReader. This ensures that the FileReader is automatically closed after its execution, even if an exception occurs.
FileReader with Encoding
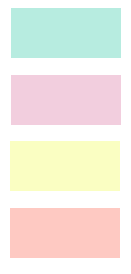
The FileReader class in Java is designed to decode bytes from a file using the default character encoding of the platform it is running on. This means that the characters read from the file are decoded based on the default character encoding of the system. However, this default character encoding may not always be suitable for all scenarios, and you cannot change it when using the FileReader class. Therefore, if you want to specify a different character decoding scheme, it is recommended to avoid using the FileReader class and use the InputStreamReader class instead.
The InputStreamReader class provides more flexibility in specifying the character encoding for decoding bytes from a file. It allows you to explicitly set the character encoding when creating an instance of the InputStreamReader. This way, you can ensure that the characters are decoded using the desired character encoding scheme.
In this example, we use the InputStreamReader class along with the FileInputStream to read characters from the file "example.txt" using the UTF-8 character encoding. By using InputStreamReader, we have control over the character encoding and can specify the desired encoding scheme explicitly.
It's important to note that when using InputStreamReader, the character encoding is cached, and changing the character encoding after creating the object will not have any effect. Therefore, it is crucial to set the character encoding at the time of creating the InputStreamReader instance. This way, you can ensure that the correct character decoding scheme is used throughout the process of reading characters from the file.
Conclusion
The FileReader class provides a straightforward way to read character data from a file, and by utilizing try-with-resources, you can ensure proper resource management and exception handling. It is essential to close the FileReader after its use to release system resources and avoid potential memory leaks.