Java String substring()
The concept of substrings holds significance when dealing with Strings. The substring refers to a portion or fragment extracted from a larger String. In Java, the String class provides a substring() method that facilitates the extraction of a specific substring from a given String.
When the substring() method is invoked, it returns a brand new String object that represents the extracted substring. This new String object is created based on the original String and contains the characters specified by the substring parameters.
There are two ways you can use the Java substring() method
- String substring(begIndex)
- String substring(begIndex, endIndex)
The substring() method in Java offers flexibility by accepting different variations of parameters. One version takes a single parameter, representing the starting index of the substring. This variant extracts the portion of the String starting from the specified index to the end of the String.
Another version of the substring() method takes two parameters, denoting the starting index and the ending index (exclusive) of the desired substring. This variant extracts the characters between the starting and ending indices from the original String.
substring(beginIndex)
The substring() method in Java, when invoked with a single parameter, allows for the extraction of a new String object starting from the specified startIndex (inclusive) and extending to the end of the original String, encompassing the last character of the String.
When using this form of the substring() method, a new String object is created, containing the characters starting from the specified startIndex and continuing until the end of the original String. The last character of the original String is also included in the extracted substring.
This functionality proves useful when there is a need to isolate a portion of a String, beginning from a specific position and extending to the end. By using the substring() method with a single parameter, developers can effortlessly obtain a new String object representing the desired substring.
Syntax- beginIndex – the beginning index, inclusive.
The above code returns String Tutorial . That means the substring function extract the new string from 5th character (start index 5 ).
Full Sourcesubstring(beginIndex,endIndex)
The substring() method in Java, when invoked with two parameters (beginIndex and endIndex), creates a new String object that includes the characters from the specified startIndex (inclusive) up to the specified endIndex (exclusive).
In other words, the character at the startIndex position is included in the extracted substring, but the character at the endIndex position is not. This exclusive nature of the endIndex parameter means that if you want to remove the last character from a String, you would use substring(0, string.length() - 1).
By specifying the beginIndex as 0 (indicating the start of the String) and the endIndex as string.length() - 1 (excluding the last character), a new String object is created without the final character, effectively achieving the desired removal.
Syntax- beginIndex – the beginning index, inclusive.
- endIndex – the ending index, exclusive.
The above code returns String , that means it extract the string from the 5th character to 11th character.
Full SourceHow to extract first 4 character from a string?
How to extract last 8 characters from a String?
How to print one char on each line?
Checking Palindrome using substring() Method
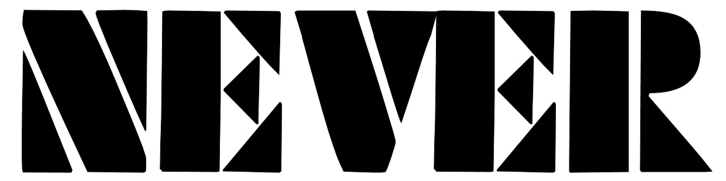
In the following Java program , checking if the first letter and the last letter of the string is same or not. If they are not the same, return false . If it is same, call the checkPal method again recursively passing the string again after the first and last letter removed.
Java StringIndexOutOfBounds Exception
When utilizing the substring() method in Java, it becomes possible to extract a specific subset of characters from a given string. It is important to note that the index values for substring operations must fall within the range of 0 to the length of the string.
However, certain conditions may lead to the occurrence of a java.lang.StringIndexOutOfBoundsException. This exception is considered an unchecked exception in Java, and it is thrown by String methods to indicate various scenarios:
If the beginIndex parameter provided is negative, indicating an index outside the valid range.
If the endIndex parameter exceeds the length of the string, signifying an index beyond the available character sequence.
If the beginIndex value is greater than the endIndex value, representing an invalid range where the start index is larger than the end index.
exampleHere you can see the length of the string is "3" and we try to extract the 4th character from the string. So, the above code would throw StirngIndexOutOfBoundException as its trying to access index 4 which doesn't exits.
Encountering a StringIndexOutOfBoundsException serves as an indication that the substring operation is being performed with invalid index values, violating the boundaries of the string. It is important to handle this exception appropriately in your code to prevent unexpected program termination and provide meaningful error messages or take corrective actions as needed.
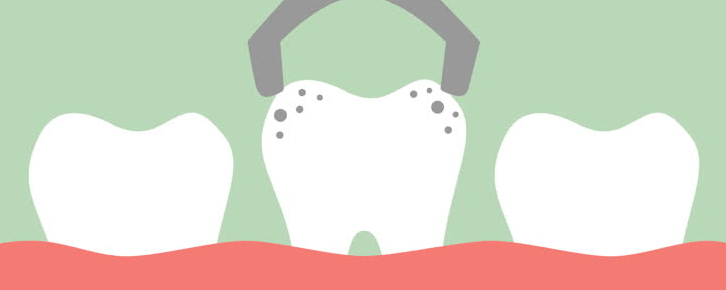
How to solve the StringIndexOutOfBoundsException
- Check the length of the string.
- Exception handling using try...catch.
Check the length of the string
You can check the length of the string using String.length() method before using subString() and proceed to access the characters of it accordingly.
Exception handling using try...catch
Using Java exception handling can be easily solved once you make use of the stack trace provided by the JVM. Once you detect the method that threw the StringIndexOutOfBoundsException , then you must examine the provided arguments are valid or not.
Conclusion
The substring() method in Java String is used to extract a portion of a string based on specified start and end indices. It returns a new string that represents the substring, including the character at the start index and excluding the character at the end index. This method provides a convenient way to work with substrings from larger strings without modifying the original string.