Async/Await in JavaScript
Async/await is a powerful feature in JavaScript used for handling asynchronous operations in a more readable and synchronous-like manner. It provides a cleaner way to work with Promises and perform asynchronous tasks without nesting callbacks. Here's a detailed explanation with examples:
Defining an Async Function
An async function is declared using the async keyword before the function declaration. This signifies that the function will contain asynchronous operations and will return a Promise implicitly.
Awaiting Promises
Inside an async function, you can use the await keyword before a Promise to pause the execution of the function until the Promise resolves or rejects. This helps avoid callback hell and promotes more linear and readable code.
Handling Errors
You can use a try/catch block to handle errors that might occur during asynchronous operations inside an async function.
Awaiting Multiple Promises Concurrently
Async/await allows you to execute multiple asynchronous operations concurrently and wait for all of them to complete using Promise.all().
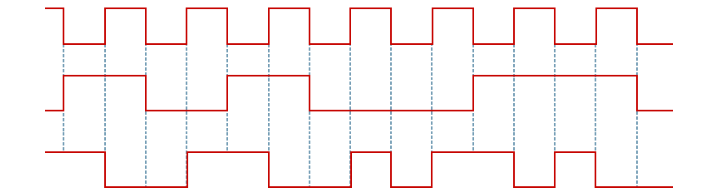
Using Async/Await with Other Promise-Based APIs
Async/await can be combined with various other Promise-based APIs, like timers, FileReader, and more.
Conclusion
Async/await greatly enhances the readability and maintainability of asynchronous code in JavaScript, making it easier to understand and manage complex asynchronous workflows. However, it's important to note that async/await is only available in modern environments (ES2017+), so be sure to check compatibility before using it.